What Is a Schema in API Development? Uses, Benefits, and Best Practices with EchoAPI
This article delves into what schemas are, their significance in API design, and how tools like EchoAPI can streamline the implementation and management of schemas to enhance API development
Schema is the official "blueprint" for your data, detailing the structure and format. It acts as a "contract" in API and service development, ensuring that everyone—front-end, back-end, devs, and testers—works with the same understanding of how data should be handled.
Think of Schema as the blueprint for your data structures—deciding which fields are mandatory, what type they should be, and whether they can be null or not.
What Does It Do?
Purpose | Explanation |
---|---|
Document Generation | Automatically generate API documentation (Swagger UI, GraphiQL, Postman) to keep docs and code in sync, eliminating the risk of mismatched documentation. |
Code Generation | Automatically generate client SDKs, backend interfaces, form validation logic, etc., to reduce manual coding and boost dev productivity. |
Frontend-Backend Alignment | Both front-end and back-end teams can develop based on the same schema, reducing communication costs and ensuring consistent data format, validation rules, and field types. |
Serialization/Deserialization | In tools like gRPC or GraphQL, Schema ensures data can be converted to the right format for cross-language/platform consistency, solving the dreaded "data transport format mismatch." |
Automated Testing & Mocking | Generate mock data based on Schema for testing APIs, helping devs conduct unit and integration tests to verify functionality. |
Data Validation | Enforce data validation rules using the Schema, ensuring that the data sent from the client matches the expected format before entering the system. |
Error Handling & Format Consistency | Standardize error responses via Schema, making error handling more predictable and easier to manage. |
Version Control & Compatibility | Track API changes with Schema versioning, supporting backward compatibility to simplify API lifecycle management and upgrades. |
Form & UI Generation | Use Schema to auto-generate forms, form validation, and UI components, reducing front-end work and ensuring back-end validation consistency. |
Dynamic Configuration & Scalability | Schema allows systems to dynamically load and adjust data structures and behaviors, reducing hardcoding and improving flexibility and scalability. |
Third-Party Service Integration | Use Schema to integrate with third-party services, ensuring a consistent data format and reducing the risk of errors during integration. |
Schema is more than just a tool for defining data structures—it plays a vital role in improving dev efficiency, automating workflows, reducing errors, and maintaining system stability.
In the API world, Schema is like the “architectural plan”—it gives data a solid structure that you can trust, measure, and control.
It defines not only the data but also the structure of the dev process itself, making things more automated, collaborative, and efficient. It's an essential part of modern service development.
Seven Benefits of Defining Schema First
Benefit | Description | Developer Pain Points Solved |
---|---|---|
Clear Frontend-Backend Contract | Schema acts as a blueprint for interfaces, defining field types and formats | Avoids missing fields, misunderstandings, and reduces communication overhead |
Automatic Code Generation | Schema can auto-generate frontend SDKs, backend validation logic, forms, and test code | Saves time on repetitive tasks and boosts delivery speed |
Strong Production | Strict verification of API data structures, with IDE support for type inference | Fewer bugs in production, better dev experience |
Auto-generated Docs | Schema auto-generates documentation for tools like Swagger, GraphiQL, and Postman | Ensures docs are always in sync with the code, simplifying external integrations |
Promotes Structure-First Development | Define the structure before logic, aligning team pace and supporting API mocks | Speeds up collaborative development and supports pre-development testing |
Supports Iteration & Version Control | Schema is versioned and easily compared, supporting backward compatibility | Simplifies API lifecycle management, especially in microservices |
Used for Testing & Validation | Auto-generate mock data, test cases, and API monitoring based on the Schema | Reduces testing costs and increases test coverage |
Example: Schema in a RESTful API
In a RESTful API, Schema is used to define data structures like request parameters, response data, and error formats. It helps developers clearly understand data format requirements, reduces errors, and auto-generates docs, code, and data validation.
Let’s take an API for user registration, where the user provides their username, email, and age. We’ll use OpenAPI 3.0 to define the Schema.
Request Example: Registering a User
You send a POST /users
request with user details. The data must match certain formats, including required and optional fields.
Request Body
{
"username": "alice",
"email": "alice@example.com",
"age": 25
}
OpenAPI 3.0 Schema Definition:
components:
schemas:
UserInput: # Schema definition for request body
type: object
required:
- username
- email
properties:
username:
type: string
description: "Username, must be a string"
email:
type: string
format: email
description: "Email address, must be a valid email format"
age:
type: integer
description: "Age, integer type, optional"
In this example, UserInput
defines the user data required for registration. We made username
and email
mandatory, while age
is optional. Each field has a type and description.
Response Example: Successful Registration
After successful registration, the system returns a response with the user's ID, username, email, and age.
Response Body
{
"id": 123,
"username": "alice",
"email": "alice@example.com",
"age": 25
}
OpenAPI 3.0 Schema Definition:
components:
schemas:
UserOutput: # Schema definition for response body
type: object
properties:
id:
type: integer
description: "Unique identifier for the user"
username:
type: string
description: "Username"
email:
type: string
format: email
description: "User's email"
age:
type: integer
description: "User's age"
Error Handling Schema Example
In a real-world RESTful API, you'll also define error responses. For example, if the user submits an invalid email or leaves a required field empty, the system returns an error message.
Error Response Example: Missing email
Field
If the email
field is missing, the system might return an error like this:
{
"error": "Missing required field",
"message": "The 'email' field is required"
}
OpenAPI 3.0 Error Response Schema Definition:
components:
schemas:
ErrorResponse:
type: object
properties:
error:
type: string
description: "Error type description"
message:
type: string
description: "Detailed error message"
In this definition, ErrorResponse
describes the structure of error info, with error
(type) and message
(details).
Full OpenAPI Example
Here’s a full OpenAPI document with request, response, and error response all in one:
openapi: 3.0.0
info:
title: User Registration API
version: 1.0.0
paths:
/users:
post:
summary: Register a user
requestBody:
description: User info
required: true
content:
application/json:
schema:
$ref: '#/components/schemas/UserInput'
responses:
'200':
description: Registration successful
content:
application/json:
schema:
$ref: '#/components/schemas/UserOutput'
'400':
description: Bad request
content:
application/json:
schema:
$ref: '#/components/schemas/ErrorResponse'
components:
schemas:
UserInput:
type: object
required:
- username
- email
properties:
username:
type: string
description: "Username, must be a string"
email:
type: string
format: email
description: "Email address, must be a valid email format"
age:
type: integer
description: "Age, integer type, optional"
UserOutput:
type: object
properties:
id:
type: integer
description: "User's unique ID"
username:
type: string
description: "Username"
email:
type: string
format: email
description: "User's email"
age:
type: integer
description: "User's age"
ErrorResponse:
type: object
properties:
error:
type: string
description: "Error type description"
message:
type: string
description: "Detailed error message"
In RESTful APIs, Schema defines and validates the structure of requests/responses, helping developers and API consumers understand data format requirements. It reduces errors and boosts dev efficiency, automatically generating docs, client code, and data validation.
Does This Seem Like a Lot of Work? Let’s Use EchoAPI!
Some features have been updated, but the overall concept remains the same.
Learn More about Using Schema in EchoAPI
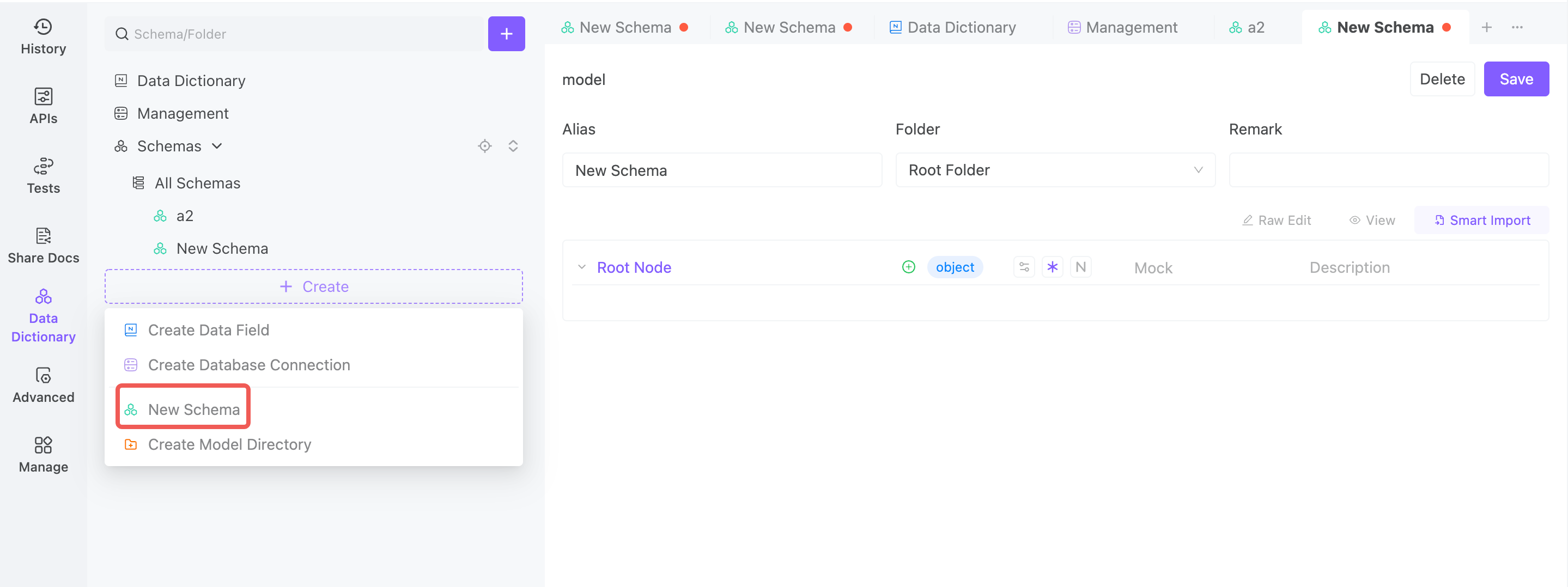
Benefits of Defining Schema with EchoAPI
1. Cut Down on Repetitive Code with Intuitive Schema Design
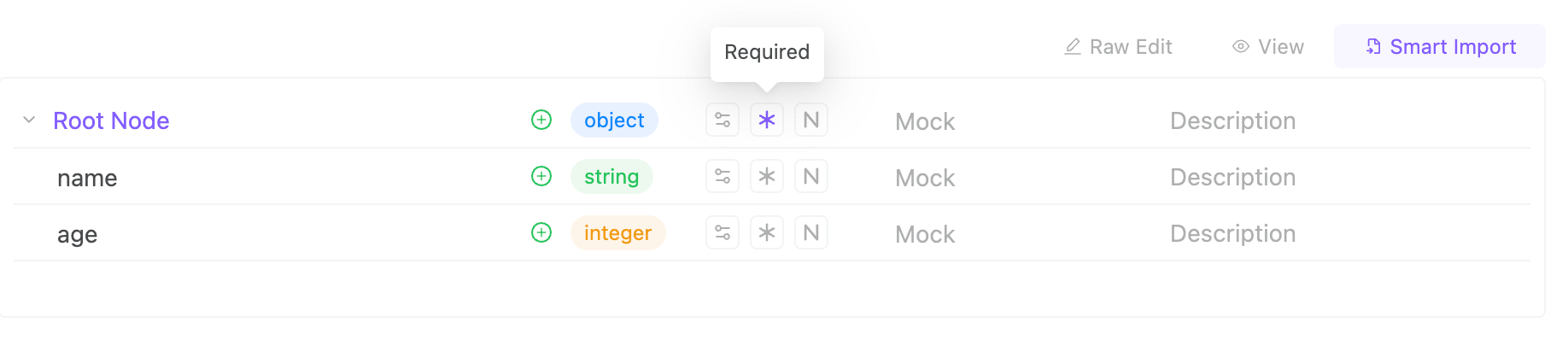
No more hand-crafting tons of repetitive code! EchoAPI provides a graphical design tool, letting devs quickly create the Schema they need with clicks, saving them from writing endless validation logic.
2. Automated Doc & Example Case Generation
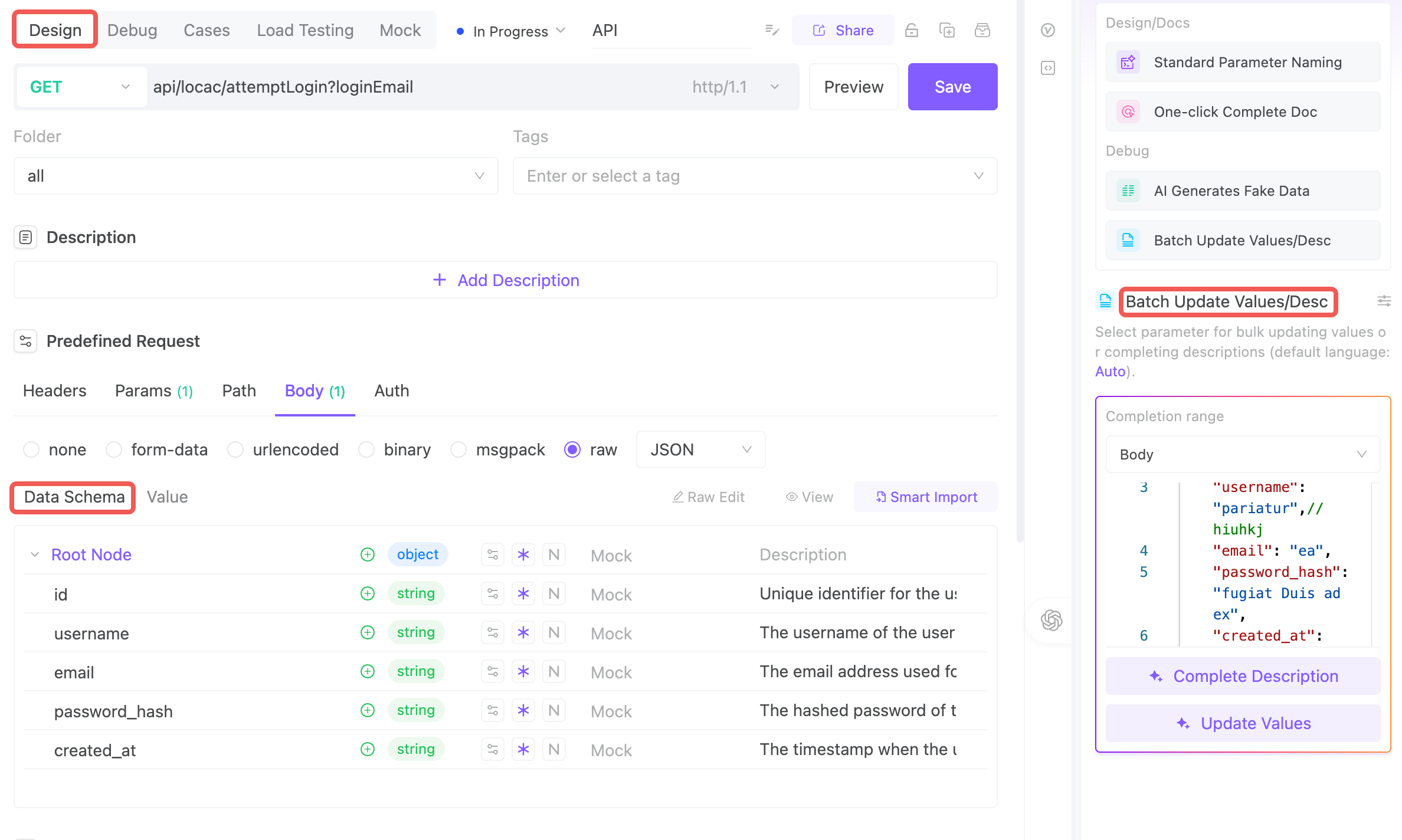
With EchoAPI, defining a Schema automatically generates your API docs and example cases. You’ll save time and avoid having mismatched docs since everything stays in sync with the code, making API integration a breeze.
3. Boost Frontend-Backend Collaboration
By using a shared Schema, front-end and back-end devs can align on data structure, reducing misunderstandings and errors. Frontend devs can clearly understand API data structures, and backend devs can make sure responses match those structures, cutting down on miscommunications.
4. Support for Multiple Formats & Flexibility
EchoAPI supports defining Schemas in JSON, XML, MySQL DDL, and more. Whether you're using JSON Schema or traditional DB table structures, EchoAPI helps you generate and apply them, increasing compatibility across different tech stacks and formats.
5. Smart Imports for Rapid Schema Generation
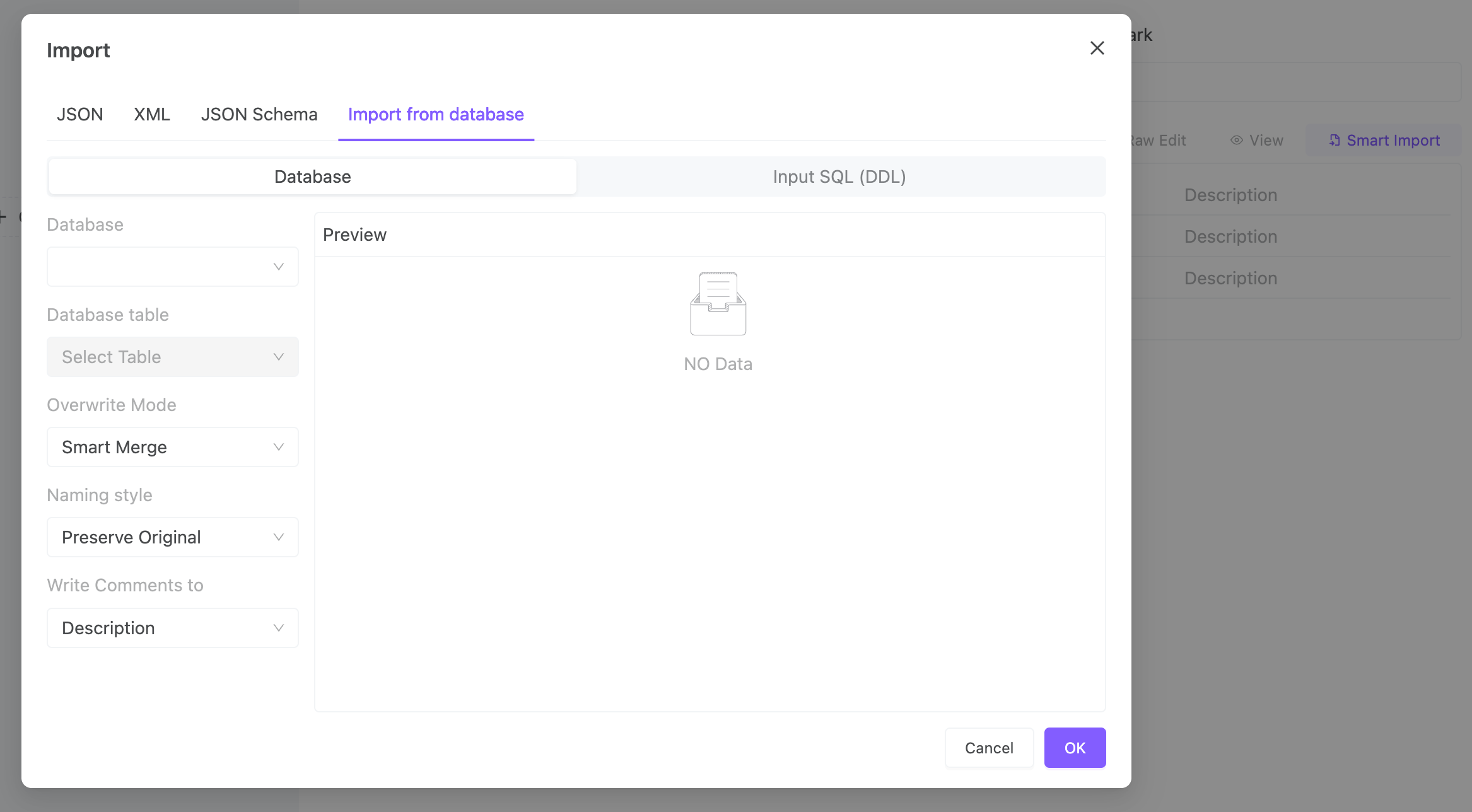
EchoAPI makes it easy to import JSON, XML, or MySQL DDL files to quickly generate your Schema. This is especially useful when you're developing based on existing databases or APIs, boosting efficiency by eliminating manual input.
6. Efficient Schema Management and Reuse
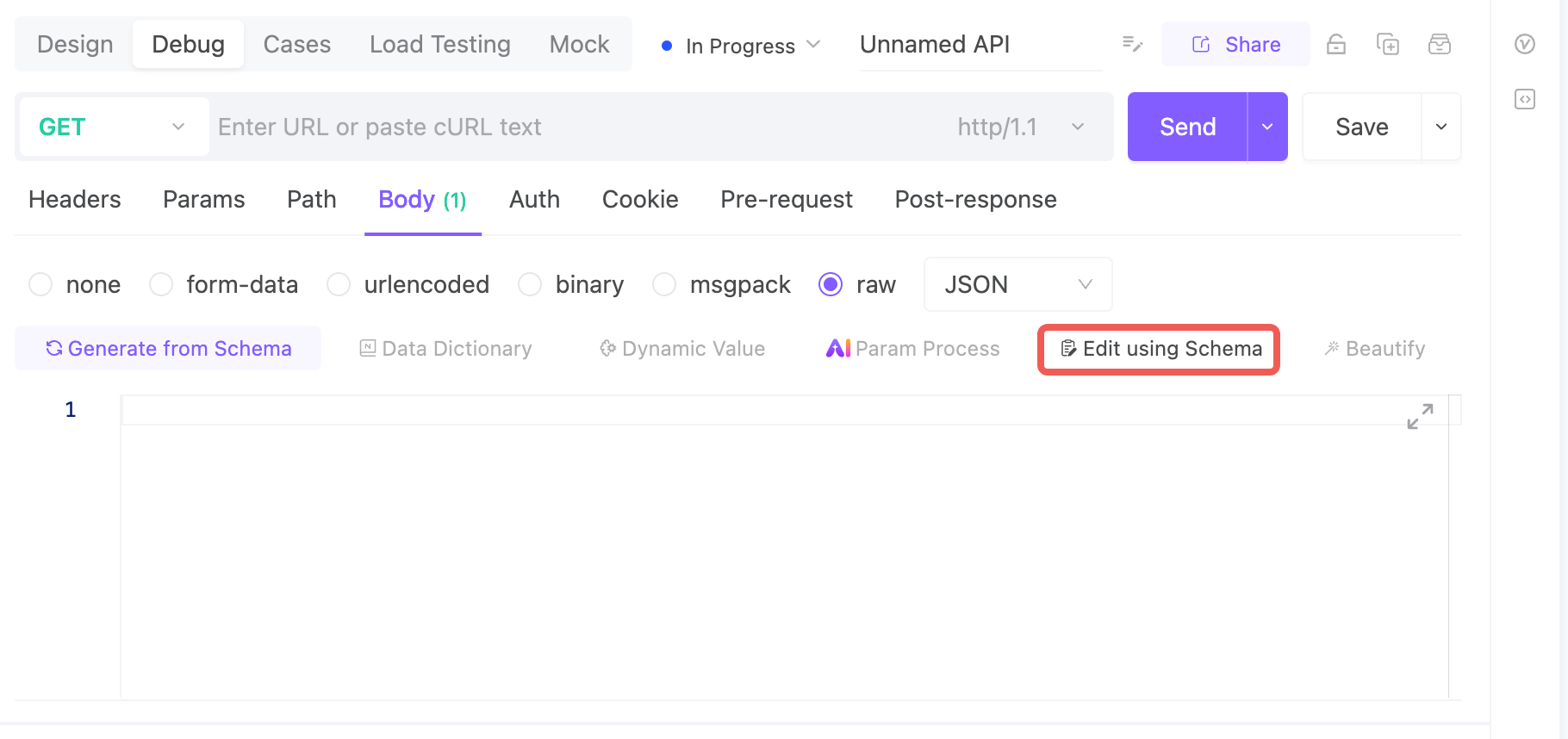
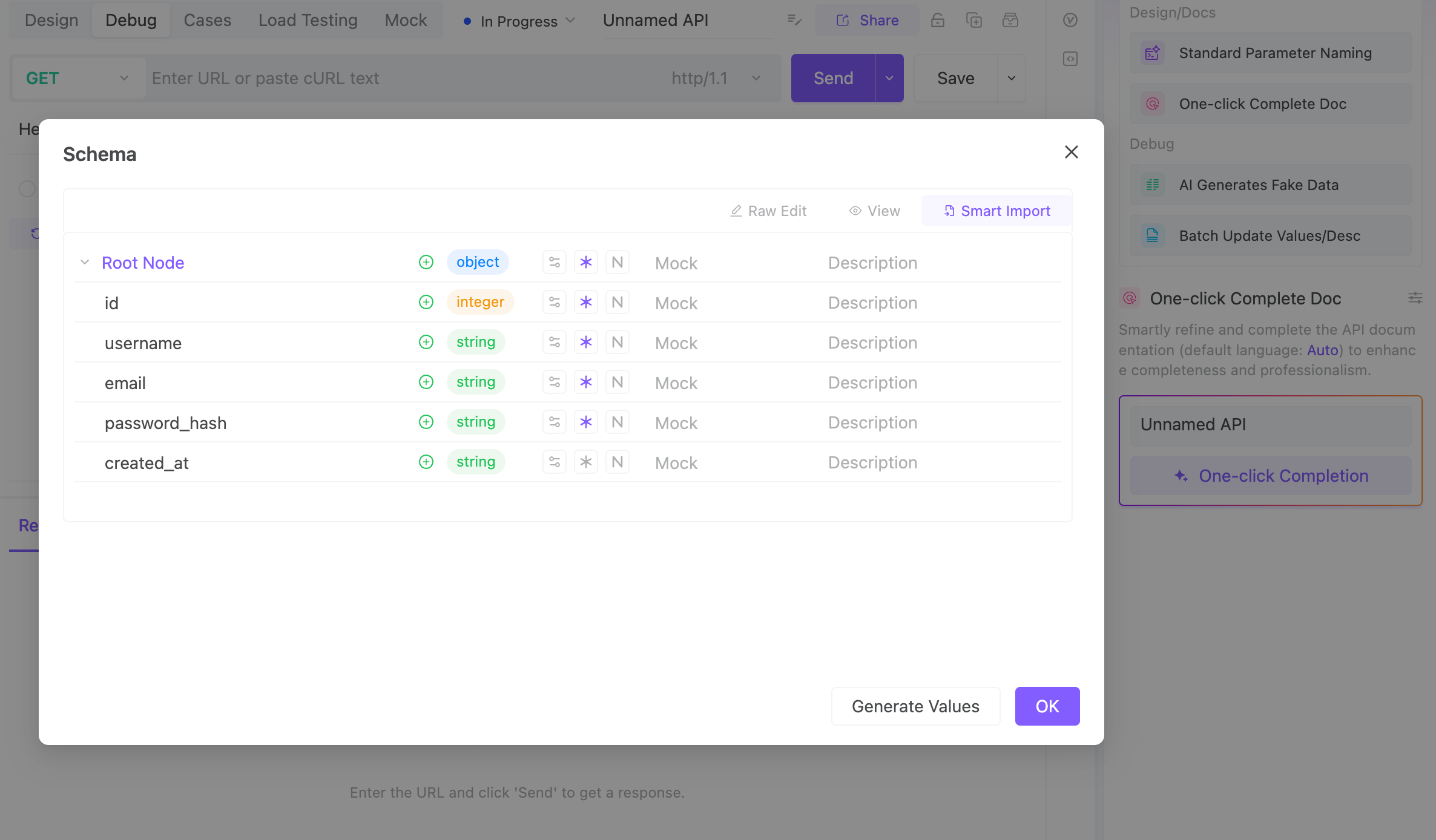
EchoAPI allows developers to categorize and reference multiple Schemas, avoiding redundant definitions. Through the referencing mechanism, common parts can be extracted into independent Schemas, simplifying the management of complex data structures, reducing duplication, and enhancing code reusability and maintainability.
7. Support for Advanced Field Settings and Customization
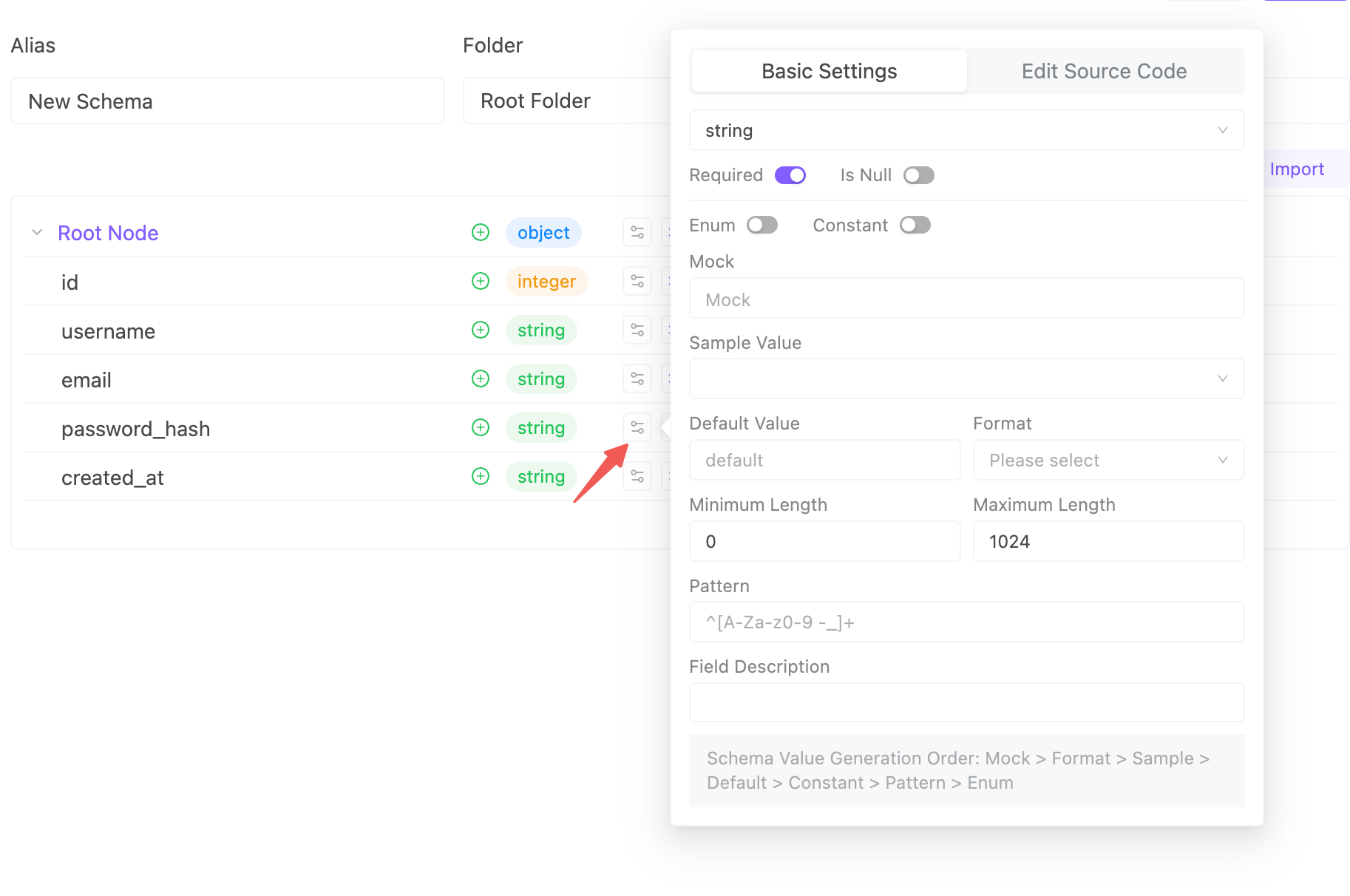
EchoAPI offers advanced field configuration options, allowing developers to set custom constraints such as array length, minimum and maximum string lengths, numeric ranges, and more. You can easily define field types, required fields, default values, and format constraints to reduce the risk of data format errors.This provides great flexibility, enabling developers to define more precise data validation rules tailored to specific business requirements, thereby ensuring structural integrity.
8. Real-time Preview and Editing Support
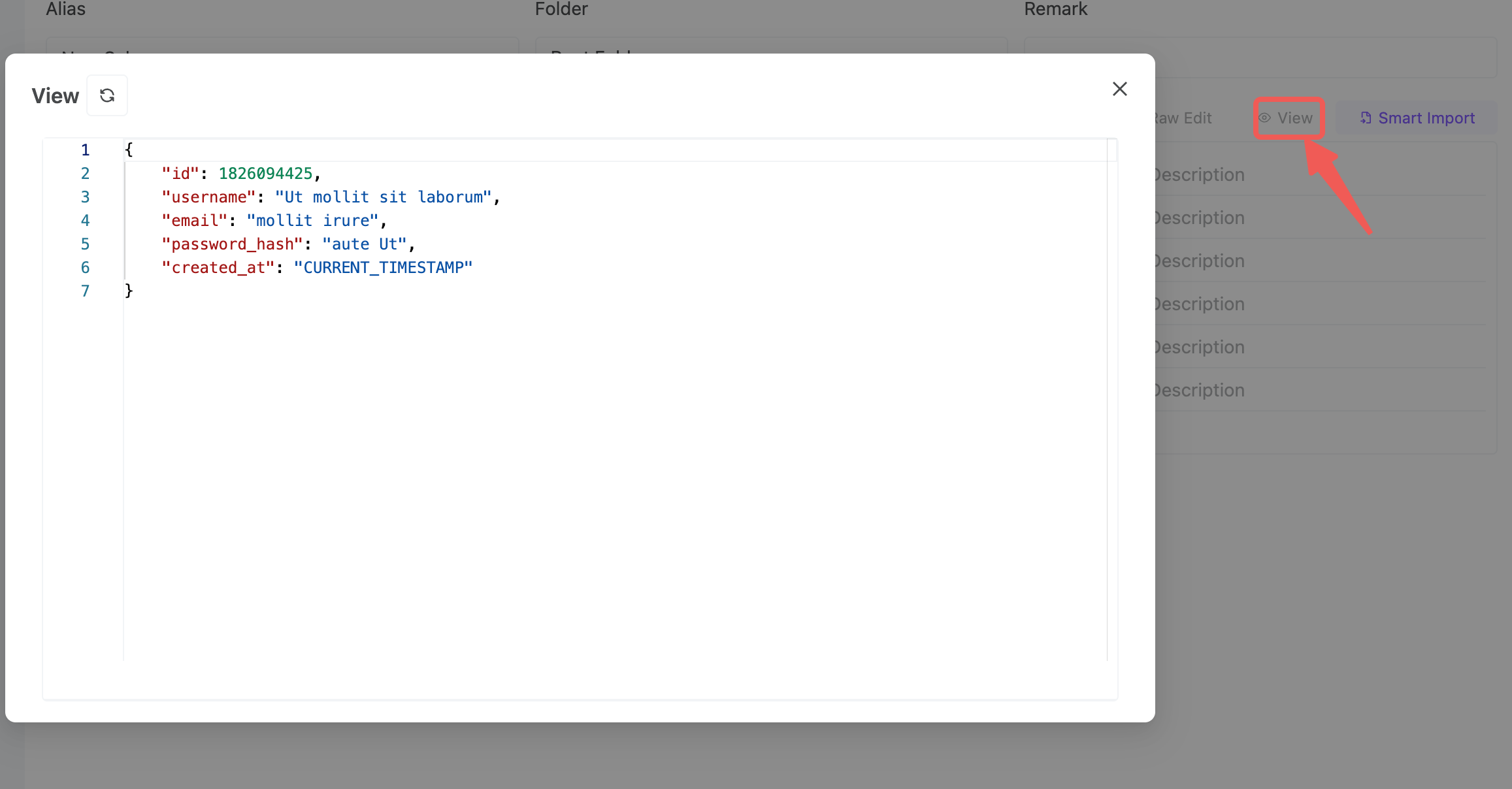
EchoAPI provides real-time preview and source code editing features, allowing developers to design Schemas visually and instantly see the effects of their changes. For cases that require fine-grained control, developers can directly edit the JSON Schema source code for greater flexibility and precision.
9. Cross-Platform Support and Tool Integration
EchoAPI is compatible with popular development tools (such as IntelliJ IDEA, VS Code) and browser plugins, enabling developers to design, debug, and test APIs within familiar environments. There’s no need to switch tools or platforms—developers can enjoy complete API management and debugging functionality seamlessly, improving both efficiency and convenience.
Conclusion
EchoAPI not only simplifies the development process but also ensures the accuracy and consistency of data structures. It helps development teams build and maintain APIs faster and more efficiently, while reducing errors that may occur during manual data structure changes.
Schema is the "contract layer" of modern API development. Whether handwritten or auto-generated, it's the core of improved collaboration, code quality, and testing assurance.