Mastering Postman: Securing Your APIs with Implementing Encryption and Decryption
The article "Mastering Postman: Securing Your APIs with Implementing Encryption and Decryption" explains the importance of encrypting APIs to protect sensitive data. It covers various encryption methods and provides a step-by-step guide on implementing them in Postman.
In today's digital landscape, where data breaches and cybersecurity threats are rampant, encryption and decryption of APIs play a crucial role in safeguarding sensitive information. This article explores why API encryption and decryption are vital, the various encryption methods available, and how you can implement these functionalities using Postman, along with an overview of the most frequently used method, MD5.
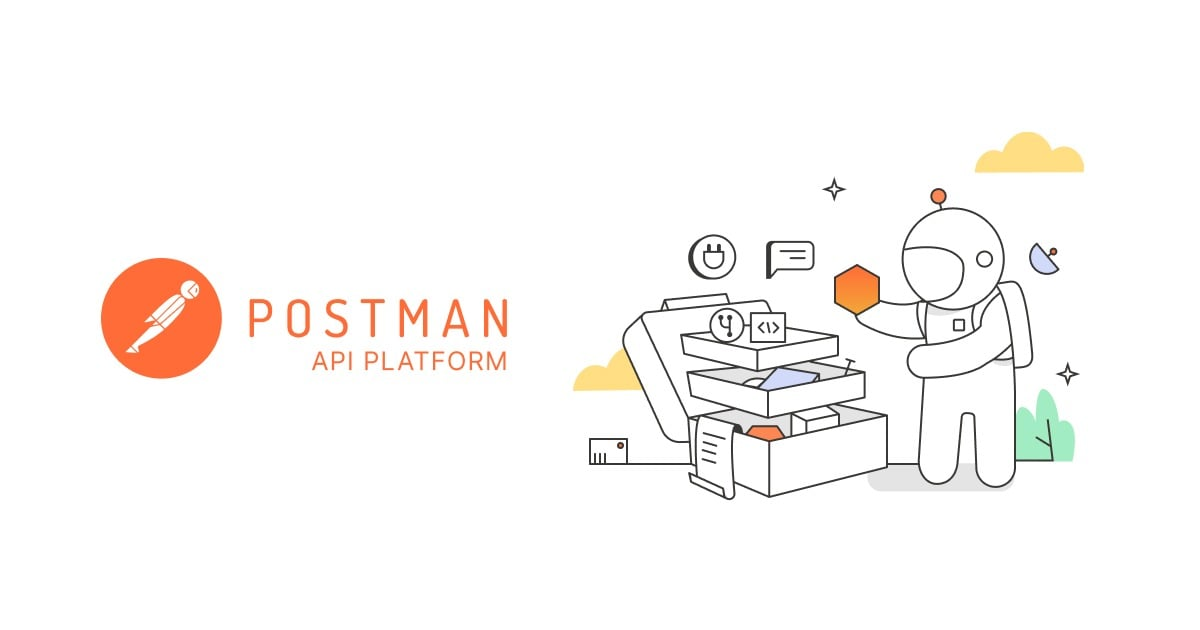
Why Encrypt and Decrypt APIs?
Encryption transforms readable data (plaintext) into an unreadable format (ciphertext) using algorithms, ensuring that only authorized parties can access the original information by decrypting it. This is particularly essential for APIs, as they often transmit sensitive information such as personal details, login credentials, and financial data. By encrypting API communications, businesses can protect data integrity, confidentiality, and authenticity, fortifying their defenses against malicious attempts to intercept and misuse information.
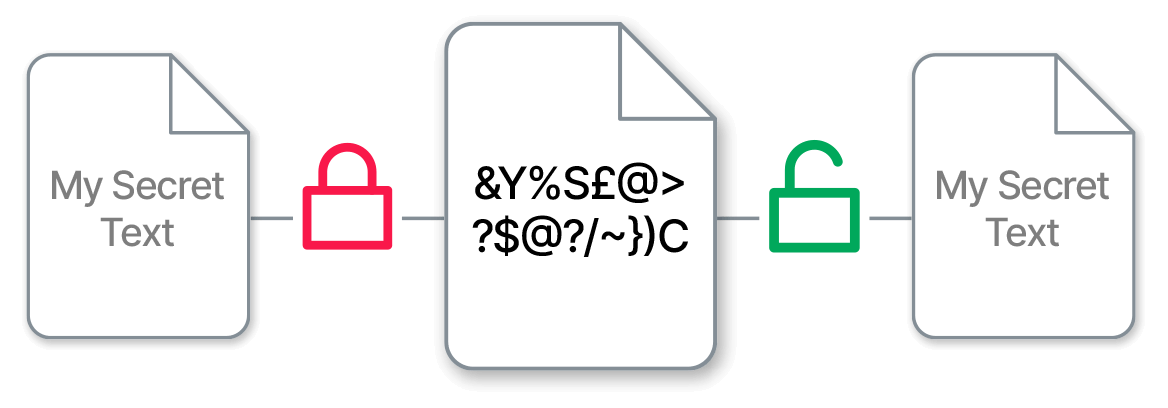
Common Encryption Methods
Symmetric Encryption
Symmetric encryption uses the same secret key for both encryption and decryption. It is efficient and suitable for encrypting large amounts of data. Common symmetric encryption algorithms include:
- DES (Data Encryption Standard): An older algorithm that uses a 56-bit key, now largely considered insecure for modern needs due to its susceptibility to brute-force attacks.
- AES (Advanced Encryption Standard): A widely used algorithm that supports various key lengths (128, 192, or 256 bits), offering strong security and performance.
- Base64: Primarily used for encoding binary data as text, not a true encryption method but often used in conjunction with other algorithms.
Asymmetric Encryption
Asymmetric encryption employs a pair of keys – a public key for encryption and a private key for decryption, or vice versa, the private key for encryption and the public key for decryption. This method is generally more secure, albeit slower than symmetric encryption, and commonly used in secure data exchanges.
- RSA (Rivest-Shamir-Adleman): The most prevalent asymmetric encryption algorithm, leveraging a pair of public and private keys, ideal for safeguarding sensitive data exchanges.
Hash Functions
Hash functions are not exactly encryption as they do not allow data to be decrypted back to its original form. Instead, they produce a fixed-size string of characters (hash) from any input size. These are typically used for data integrity checks.
- MD5 (Message-Digest Algorithm 5): Widely used for checksum and integrity verification, though not recommended for security-critical applications due to vulnerability to collision attacks.
- SHA1/SHA3: More secure hash algorithms, with SHA3 being the recent addition offering higher levels of security.
Implementing Encryption and Decryption in Postman
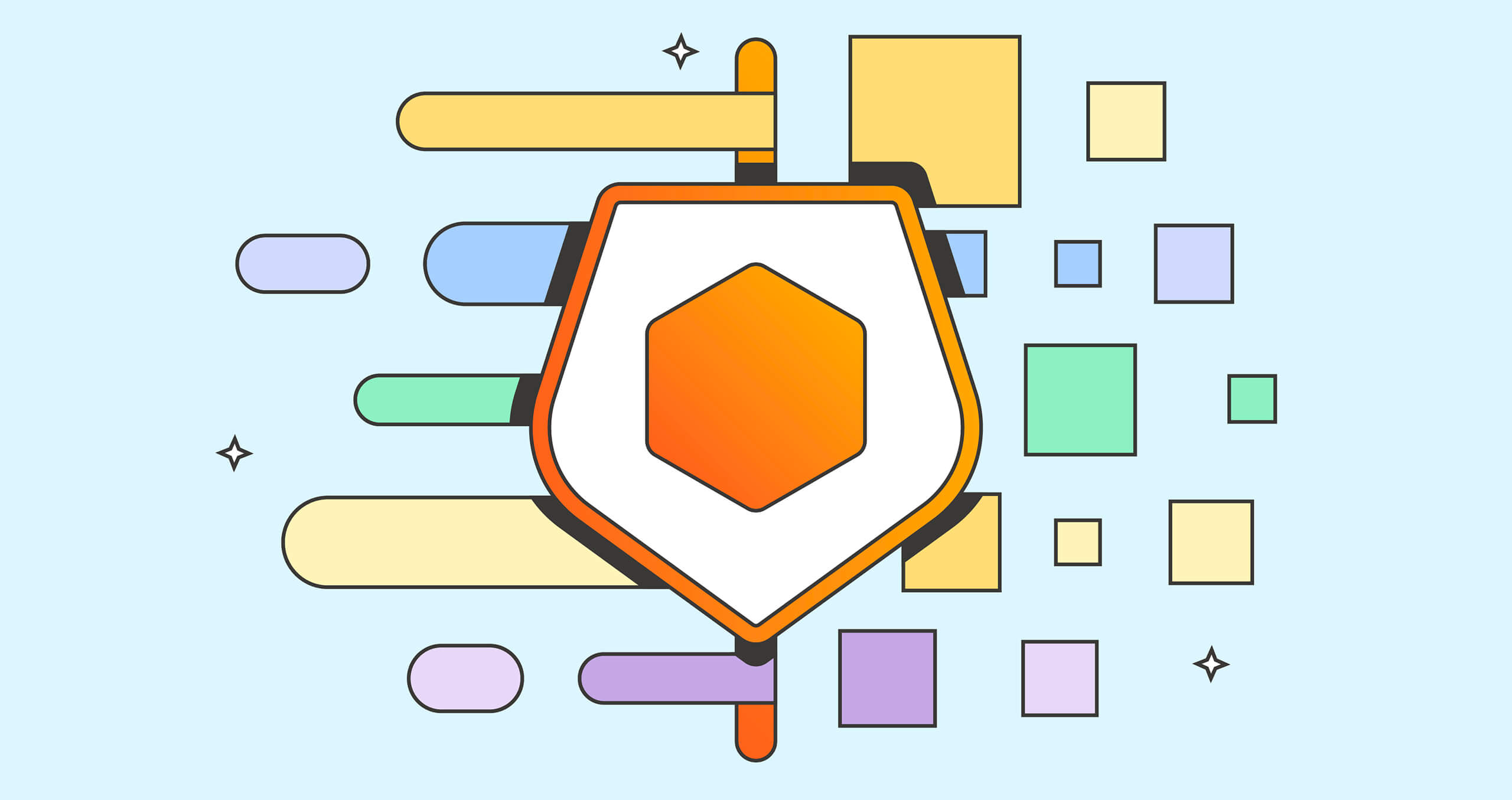
Postman provides a user-friendly interface to implement API encryption and decryption, facilitating secure data handling:
Step-by-Step Guide:
- Set Up Your Request: Configure your API request as usual, choosing the endpoint and method.
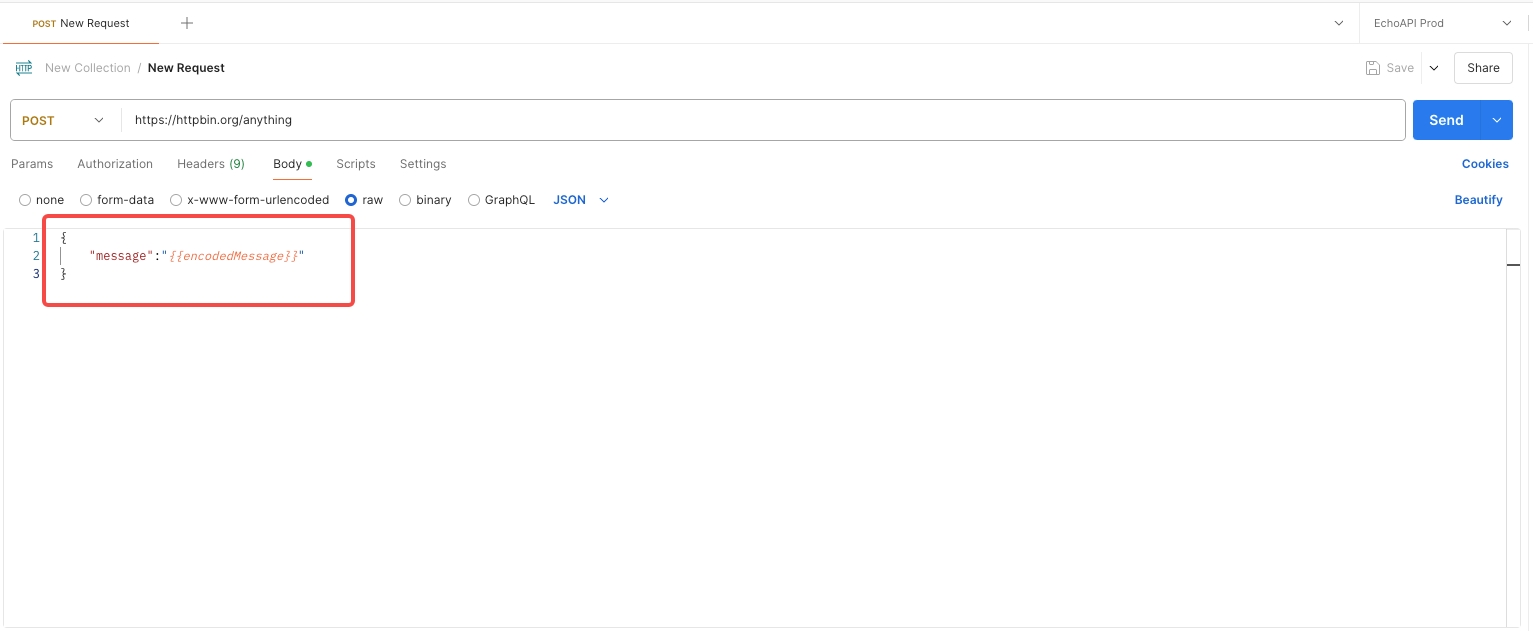
Encrypt Data: Use a Pre-request Script to encode data before sending it. For example, encrypt a payload using Base64:
let message = 'Your secret message';
let encodedMessage = require('btoa')(message);
pm.environment.set('encodedMessage', encodedMessage);
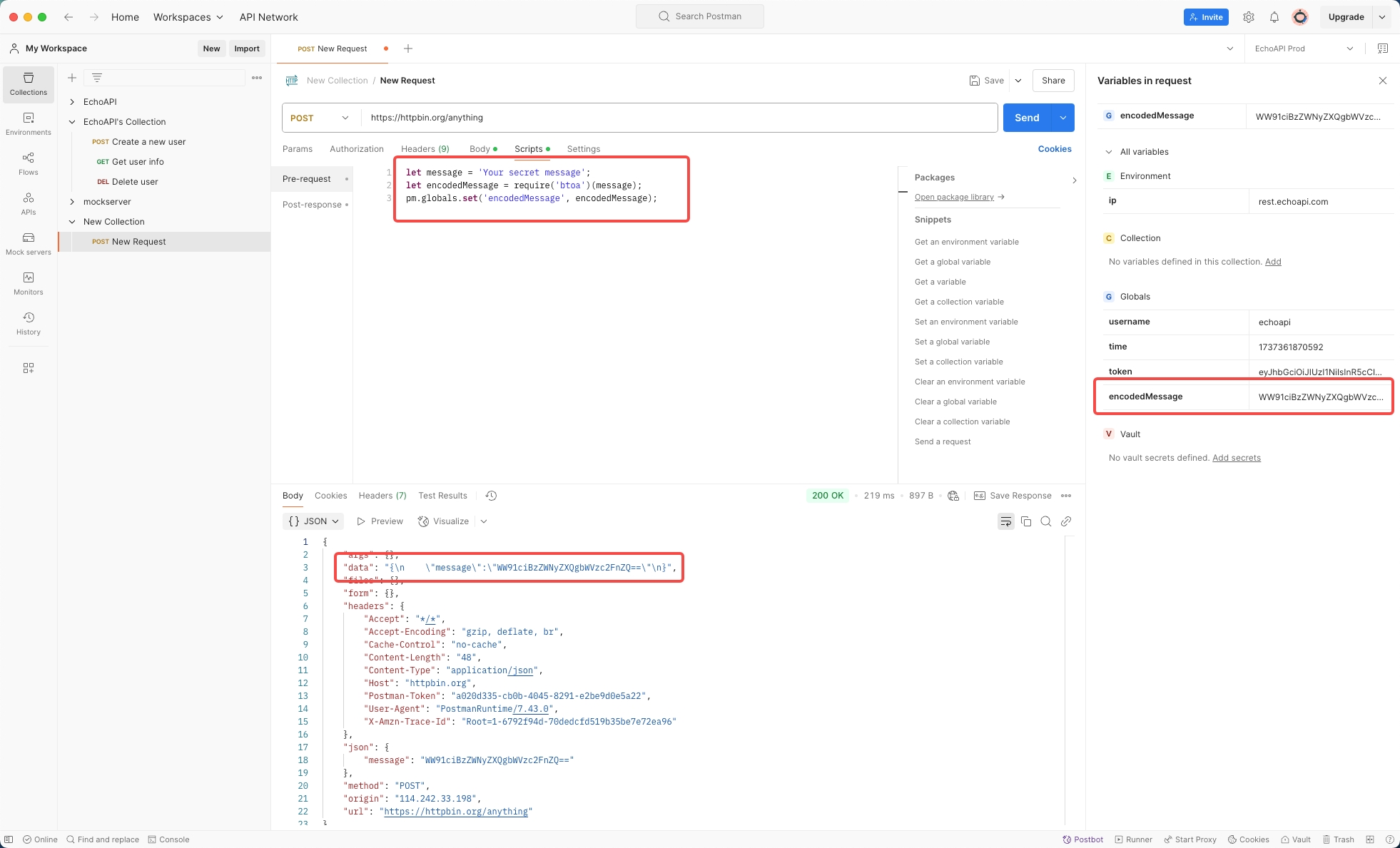
Decrypt Data: Use a Test Script to decode data after receiving the response. For example, decode a Base64 encoded message:
let encodedMessage = pm.environment.get('encodedMessage');
let decodedMessage = require('atob')(encodedMessage);
console.log(decodedMessage);
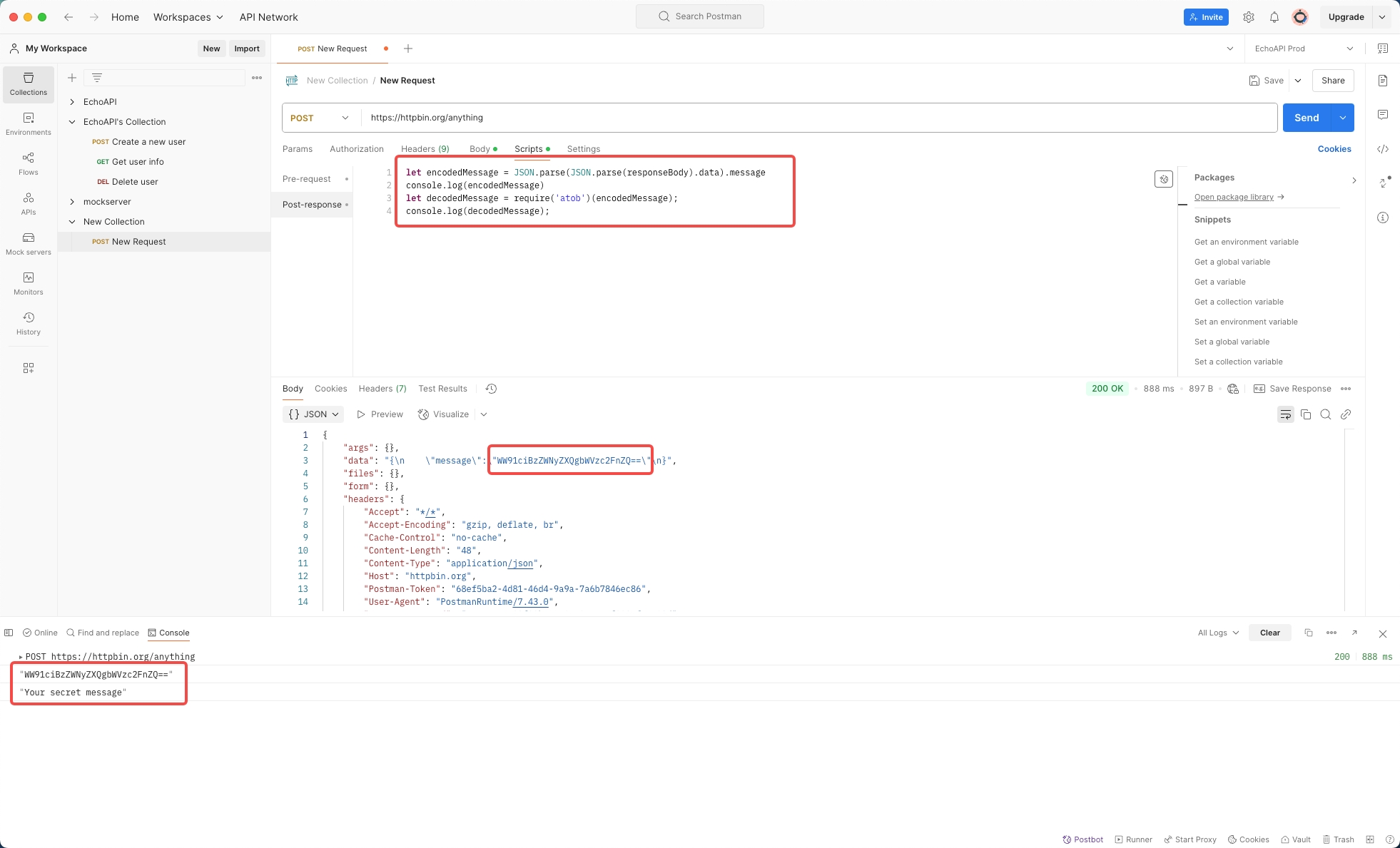
Example Using MD5
While MD5 is not used for encryption but for checksums or fingerprinting data, it can be implemented in Postman for verification purposes:
let cryptoJs = require('crypto-js');
let message = 'Your secret message';
let encodedMessage = cryptoJs.MD5(message).toString();
pm.environment.set('encodedMessage', encodedMessage);
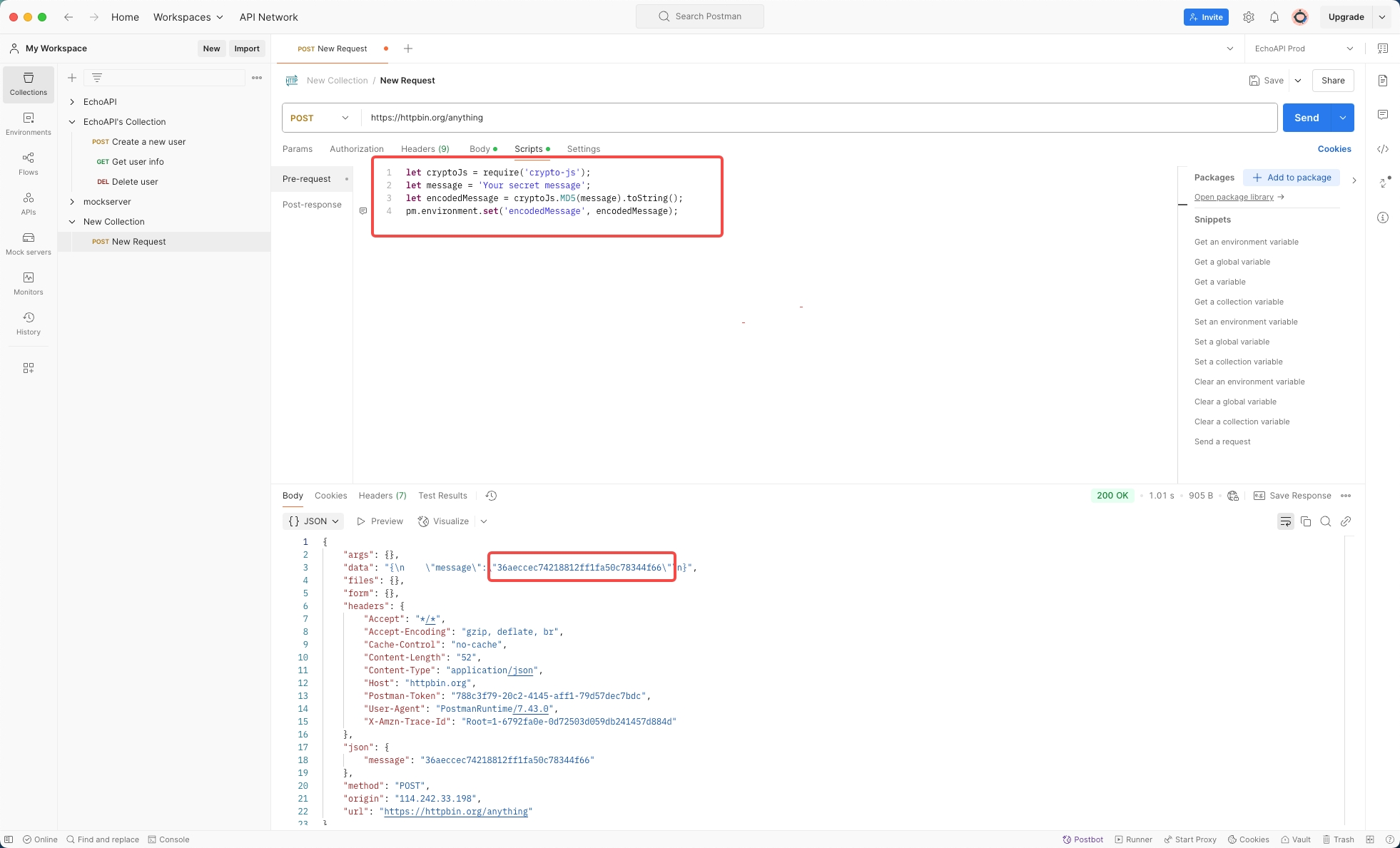
Conclusion
Encryption and decryption are essential components for securing APIs and protecting sensitive information from unauthorized access. Various methods, such as symmetric and asymmetric encryption, as well as hash functions, offer solutions tailored to different security needs. Postman, with its robust scripting capabilities, allows developers to implement and test these encryption strategies efficiently.
Utilizing these techniques ensures that data transmitted via APIs remains confidential and tamper-proof, contributing to an overall secure application environment. As threats evolve, staying ahead with effective encryption strategies becomes not just a best practice, but a necessity for modern API security.