Master API Protocols with EchoAPI: Your Debugging Savior
In the chaotic battlefield of API protocol debugging, EchoAPI emerges as the ultimate weapon. This article delves into the intricacies of various API protocols and illustrates how EchoAPI can streamline your debugging process, enhance efficiency, and transform the way you handle API development.
What's the worst nightmare for a backend dev?
Spoiler alert: it's not writing code.
It’s debugging APIs, fighting protocols, running tests, and getting that dreaded ping from frontend:
“Hey... your API broke again.”
Why?
Because there are too many protocols, too many tools, too many edge cases, and not enough time to hand-code every little thing.
Why Are There So Many API Protocols?
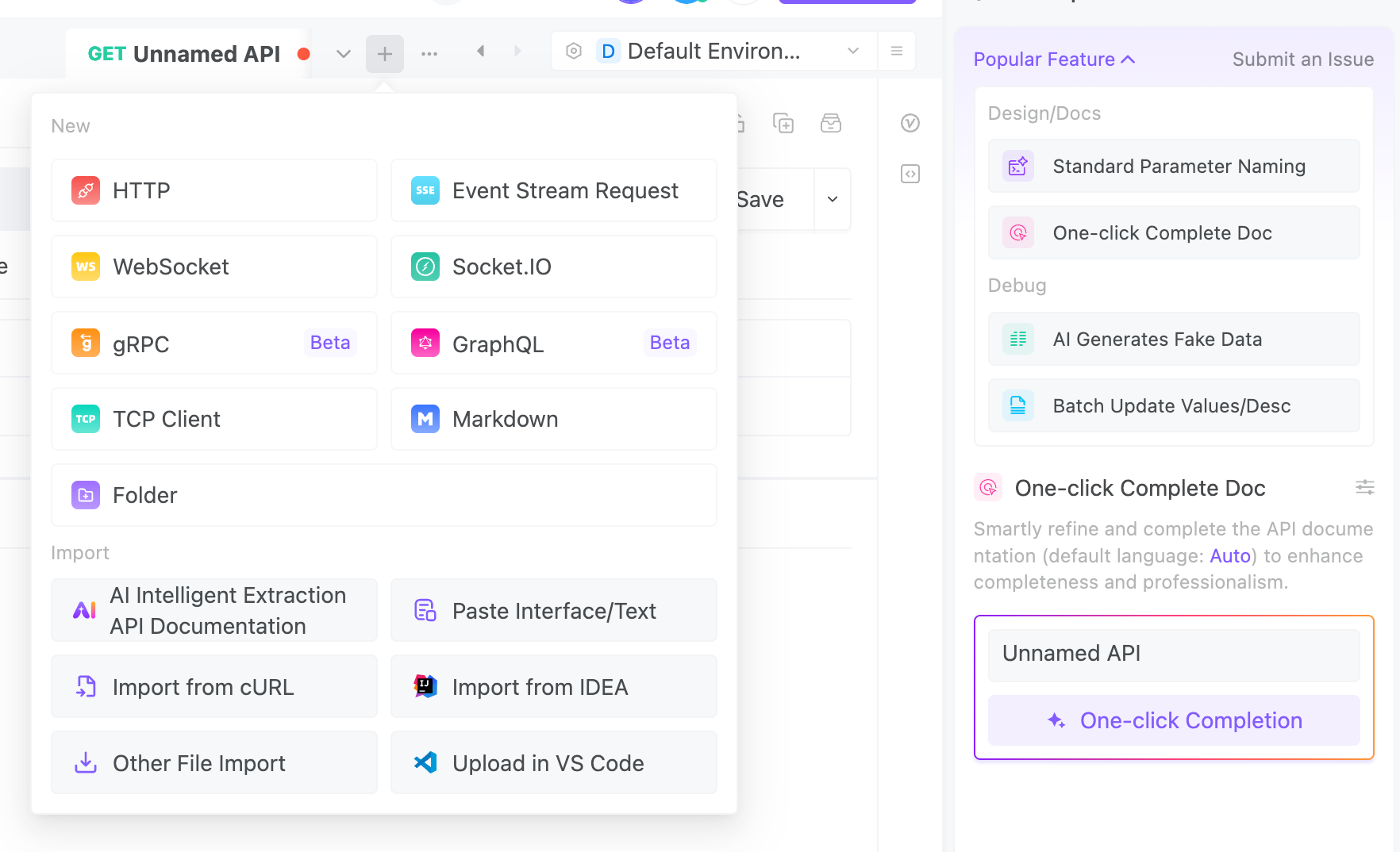
Do we really need all of them?
Yes!
Because different business needs call for different priorities — speed, bi-directional flow, security, flexibility.
You don’t bring a spoon to a sword fight. And a Swiss Army knife can’t just be... a screwdriver.
Let’s break down the most common API protocols, what they’re great at, and how they’ve helped us (or betrayed us):
1. HTTP / RESTful API — The OG of web APIs
âś… Features
- Request-response model with familiar verbs:
GET
,POST
,PUT
, etc. - Resource-based URLs. Clean and semantic.
- Caching-friendly. Easy to debug.
- Fully supported by browsers, and of course, by EchoAPI.
âś… Pros
- Simple. Every dev knows it.
- Tons of tooling support.
- Works great with frontend frameworks (HTML, JS, React, you name it).
Real-world Example
You're checking out on Amazon:
- Click "Buy Now" →
POST /orders
- View your orders →
GET /orders
Ideal For
E-commerce, CRUD systems, search/filter/query endpoints.
Solves
Gives structure and predictability. Frontend devs don’t need to care about internals — just the path and params.
⚠️ Gotchas
- Repetitive boilerplate
- Easy to mess up documentation
- Status codes are a minefield
2. WebSocket — Real-time, full-duplex communication
âś… Features
- Persistent connection. Client and server can push messages to each other.
- No HTTP header bloat. Faster messaging.
- One handshake, many chats.
âś… Pros
- Ultra-low latency.
- Perfect for real-time updates.
- Message format? Anything goes — JSON, binary, etc.
Real-world Example
You’re chatting on WhatsApp Web. Messages appear instantly without refreshing the page.
Ideal For
Chat apps, stock tickers, multiplayer games, live dashboards.
Solves
Breaks HTTP’s one-way limitation: finally, the server can talk first.
⚠️ Gotchas
- Connection state management is tricky.
- Testing and debugging can be a pain.
3. Socket.IO — WebSocket with Superpowers
âś… Features
- Wraps WebSocket with fallback (polling), reconnect logic, heartbeats.
- Built-in rooms, namespaces, events.
- Mature libraries for both frontend & backend.
âś… Pros
- Super compatible (even if WebSocket isn’t).
- Business logic friendly (supports events, channels).
- Easier frontend integration.
Real-world Example
You're in a League of Legends match with live voice chat and real-time updates.
Ideal For
Online collaboration tools, real-time games, live quizzes, webinars.
Solves
No need to reinvent wheels for reconnects, broadcasting, or message routing.
⚠️ Gotchas
- Same as WebSocket: state is hard, and debugging is tougher than REST.
4. gRPC — The microservices whisperer (by Google)
âś… Features
- Based on HTTP/2, supports multiplexing and streaming.
- Strongly typed, auto-generates client & server code.
- High performance, low overhead.
âś… Pros
- Faster and lighter than REST.
- Enforces strict API contracts.
- Works across multiple languages.
Real-world Example
You're using your mobile banking app to check your balance — behind the scenes, it’s calling a chain of microservices via gRPC.
Ideal For
Microservice communication, banking systems, IoT networks.
Solves
REST + JSON is too bloated for internal service calls. gRPC is lean, strict, and fast.
⚠️ Gotchas
- Debugging isn't trivial.
- Updating proto files across services takes planning.
5. GraphQL — APIs meet buffet: take what you need
âś… Features
- Clients specify exactly what fields they want.
- Fetch multiple resources in one go (bye-bye N+1).
- Single endpoint for everything.
âś… Pros
- Saves bandwidth. Frontend-friendly.
- Encourages reuse.
- Eliminates over-fetching/under-fetching.
Real-world Example
You open a news app that loads thumbnails, titles, authors, and suggested articles — all in one smart query.
Ideal For
Content-heavy apps (like Medium, YouTube, Pinterest).
Solves
REST is too coarse. GraphQL puts the frontend in control of the data it needs.
⚠️ Gotchas
- Caching is tough.
- Access control is harder.
- Higher learning curve.
6. SSE / EventStream — Server-to-client push, the simple way
âś… Features
- Uses HTTP
- Text-based push stream (
EventStream
) - Auto-reconnect support
âś… Pros
- Easier than WebSocket
- Browser-native support
- Lightweight and readable
Real-world Example
You're on an eBay flash sale, and the "Only 20 left!" counter updates live without a page refresh.
Ideal For
Lightweight dashboards, admin panels, real-time notifications.
Solves
A sweet spot between polling and WebSocket for pushing live data to clients.
⚠️ Gotchas
- Not supported in all browsers.
- Hard to manage client state.
7. TCP / Custom Protocols — When you need raw power
âś… Features
- Bare metal — no abstraction
- Totally customizable packet format
- Often used for binary data and extreme performance
âś… Pros
- Absolute speed
- Bit-level control
- No protocol baggage
Real-world Example
You’re playing Call of Duty. Bullet hits and player movements need millisecond accuracy — handled by raw TCP.
Ideal For
High-frequency trading, industrial sensors, MMO games.
Solves
Sometimes HTTP is too slow or bloated. TCP + custom protocols = surgical performance.
⚠️ Gotchas
Debugging basically means drowning in logs and juggling Wireshark like a circus act...
Why Does EchoAPI Support So Many Protocols?
And why should backend devs care?
As backend engineers, we’ve all been there:
"Testing protocol X with curl, Y with Postman, Z with my custom script… while trying not to cry."
Before EchoAPI, I had 3 terminals open, a bunch of cURL commands, some half-broken docs, and zero patience.
Now?
EchoAPI boosted my API testing productivity by 20x.
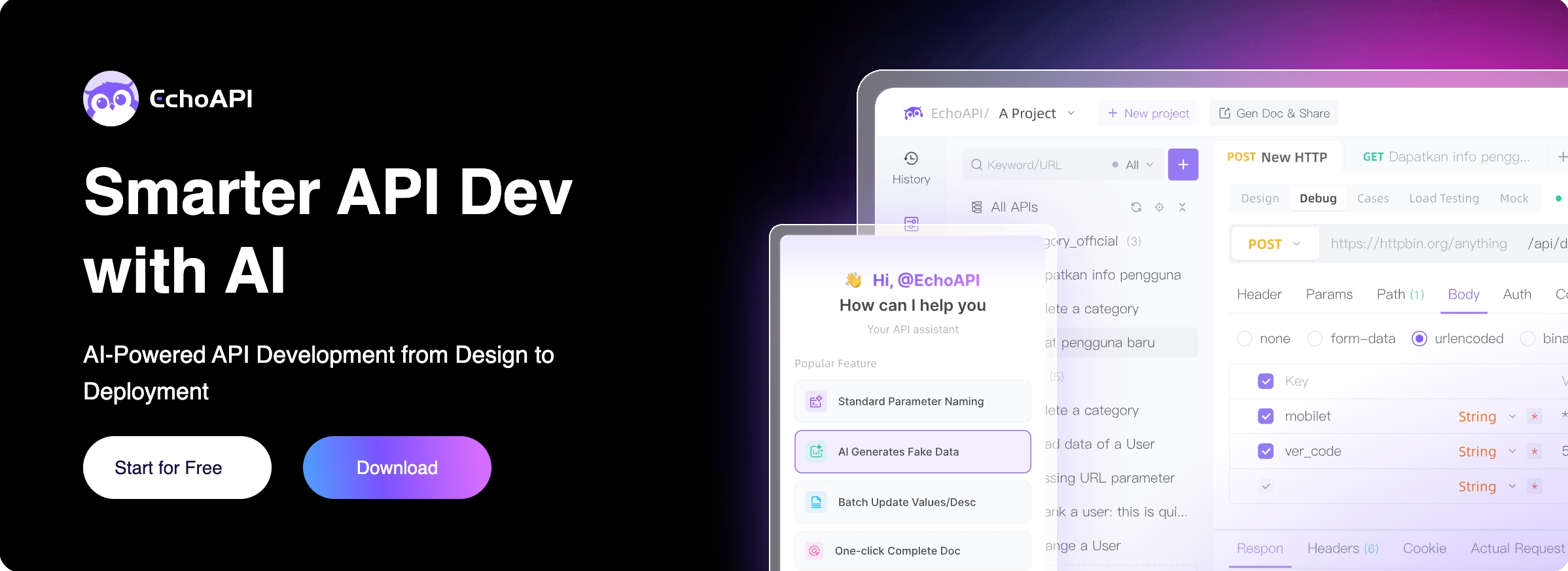
1. One Tool to Test Them All
REST, WebSocket, gRPC, GraphQL — no more juggling 6 tools. EchoAPI handles it all in one place.
2. Multi-protocol integration just works
- WebSocket? Plug in and test.
- gRPC? Upload your proto and go.
- GraphQL? EchoAPI autoloads schema with autocomplete.
3. Full HTTP Method Support (even the weird ones)
Beyond GET
and POST
, it supports PUT
, PATCH
, DELETE
, HEAD
, OPTIONS
— and even business-heavy but browser-ignored ones like COPY
, LOCK
, PROPFIND
, PURGE
, VIEW
.
4. Auth, Headers, Tokens? No Problem.
Test logged-in APIs without shell scripts or browser hacks.
5. Save, reuse, and automate tests like a pro
EchoAPI lets you save calls, rerun test suites, and even export documentation. Goodbye to Postman + JMeter + a mess of notes.
TL;DR
With EchoAPI, I get to focus on logic — not on how the heck I’m supposed to call the API.
Yes, protocols are complex.
But EchoAPI makes them visible, testable, and understandable.
It’s not just a tool — it’s your personal:
- Protocol radar
- Debugging assistant
- API doc machine
And the reason you can finally enjoy API work again.
Supporting all these protocols isn't a gimmick — it's a necessity.
Each one has a job, and EchoAPI is the one boss that knows how to handle them all.