How to Send POST Requests in Python: A Beginner's Guide
Learn how to send POST requests in Python with this beginner-friendly guide covering setup and best practices.
Learn how to send POST requests using Python. This guide covers everything from setting up your environment to best practices for secure and effective API communication.
In this article, you'll explore the fundamentals of POST requests, how to send them using Python's Requests library, and best practices to keep in mind. Whether you're an experienced developer or a beginner, this guide provides all the essential information you need about POST requests.
1. Understanding the Basics of HTTP Requests
Before diving into POST requests, it's important to understand what an HTTP request is. HTTP (Hypertext Transfer Protocol) is a protocol used to transfer data over the internet. An HTTP request is a message sent from a client to a server, requesting a specific resource. The server then responds with the requested resource.
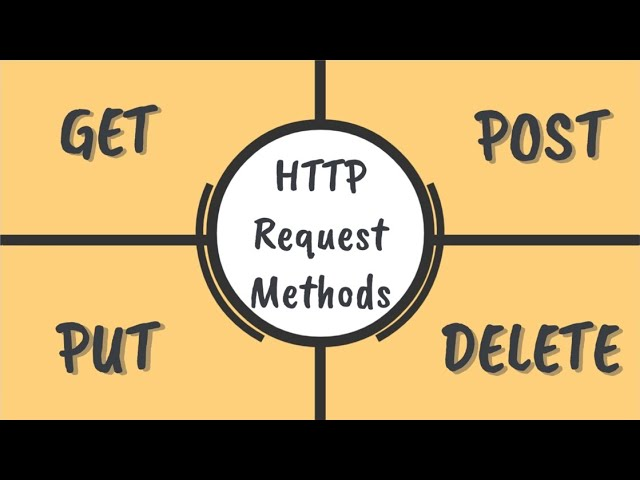
There are various types of HTTP methods, each serving different purposes and conveying the nature of the request. The most common HTTP methods include GET, POST, PUT, and DELETE.
2. What is a POST Request?
A POST request is an HTTP method used to send data to a server to create or update a resource. Unlike GET requests, where data is included in the URL, data in POST requests is included in the body of the request. This method is commonly used for submitting form data or uploading files.
3. What is Python?
Now that you've learned the basics of HTTP requests, let's introduce the Python programming language. Python is renowned for its simplicity, readability, and flexibility, enabling developers to write clear and logical code for projects of any size. You can download the latest version of Python from the official website.
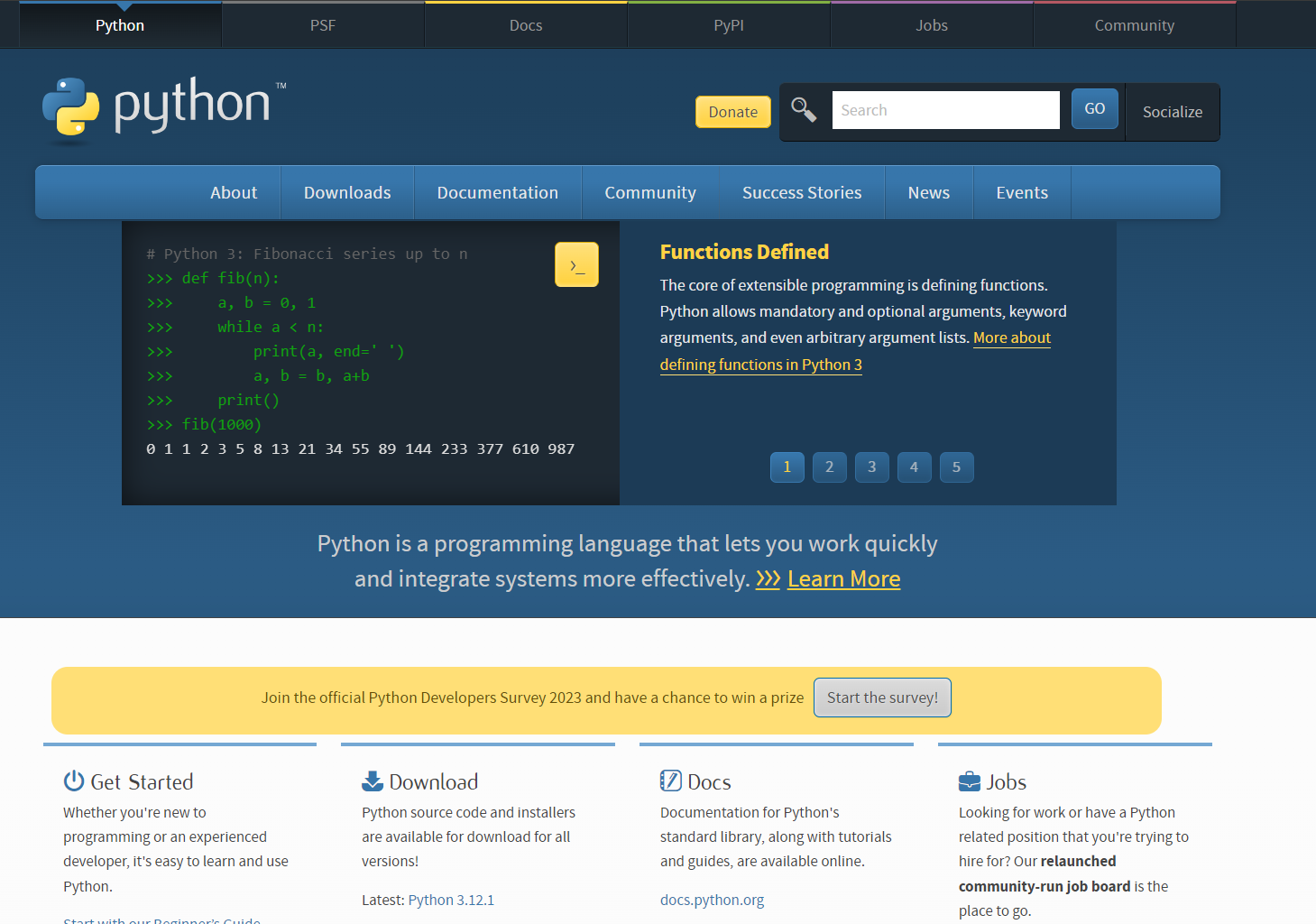
Python's extensive libraries are popular among developers, and its syntax allows even beginners to quickly grasp the concepts.
4. How to Perform a POST Request in Python
To perform a POST request in Python, you can use the popular HTTP library requests
. Here's an example of a POST request:
import requests
# Define the URL and data to send
url = 'http://example.com/test/demo_form.php'
data = {'name1': 'value1', 'name2': 'value2'}
# Make the POST request
response = requests.post(url, data=data)
# Print the status code and response content
print(f"Status Code: {response.status_code}")
print(f"Response Content: {response.text}")
In this code snippet, two pieces of data (name1: value1
and name2: value2
) are sent to http://example.com/test/demo_form.php
. The requests.post
method sends the POST request, and the server's status code and response content are printed out.
To run this code, you need to have the requests
library installed. You can install it using the following command:
pip install requests
5. Understanding POST Request Parameters in Python
When using the requests
library in Python to perform a POST request, you'll primarily deal with the following parameters:
- url: The URL to which the POST request is sent.
- data: The data you want to send in the request body (can be a dictionary, list of tuples, bytes, or file objects).
- json: A JSON object to send in the request body.
Here's an overview of how to use these parameters:
import requests
# URL for the POST request
url = 'http://example.com/api'
# Data to send in the POST request body
data = {
'key1': 'value1',
'key2': 'value2'
}
# Make the POST request
response = requests.post(url, data=data)
# Check the response
print(response.text)
In this example, data
is a dictionary containing the data to be sent to the server. If you want to send JSON data, use the json
parameter instead, which automatically sets the Content-Type
to application/json
.
Additionally, the requests.post
function accepts other keyword arguments (**kwargs), such as:
- headers: A dictionary of HTTP headers to send with the request.
- cookies: A dictionary of cookies to send with the request.
- files: A dictionary of files to send with the request.
- auth: A tuple to enable HTTP authentication.
- timeout: The number of seconds to wait for the server to send data before giving up.
- allow_redirects: A boolean indicating whether to allow POST redirects.
These parameters allow you to customize your POST requests to meet the server's requirements.
6. Testing Python POST Requests with EchoAPI
EchoAPI is a powerful tool for API testing. It allows you to create and save API requests, organize them into collections, and share them with your team.
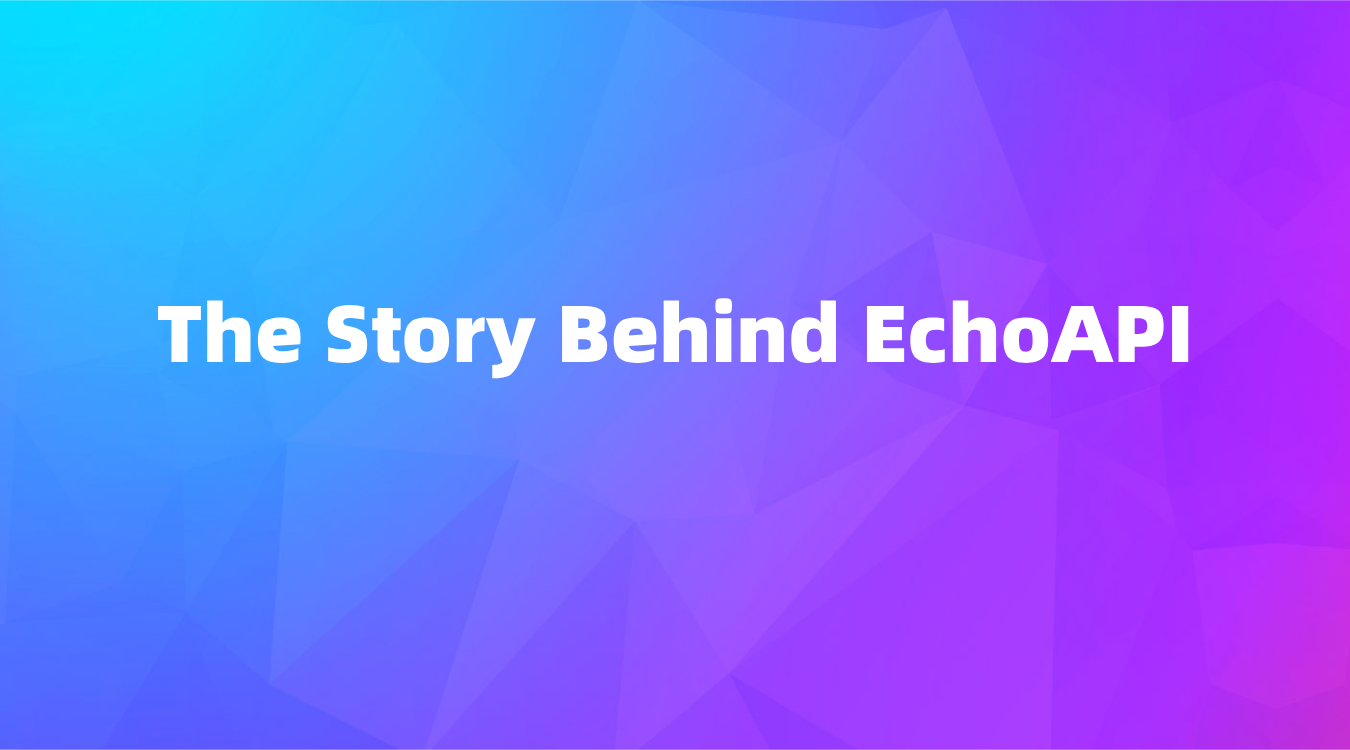
Here's how to test a POST request using EchoAPI:
1. Open EchoAPI and Create a New Request
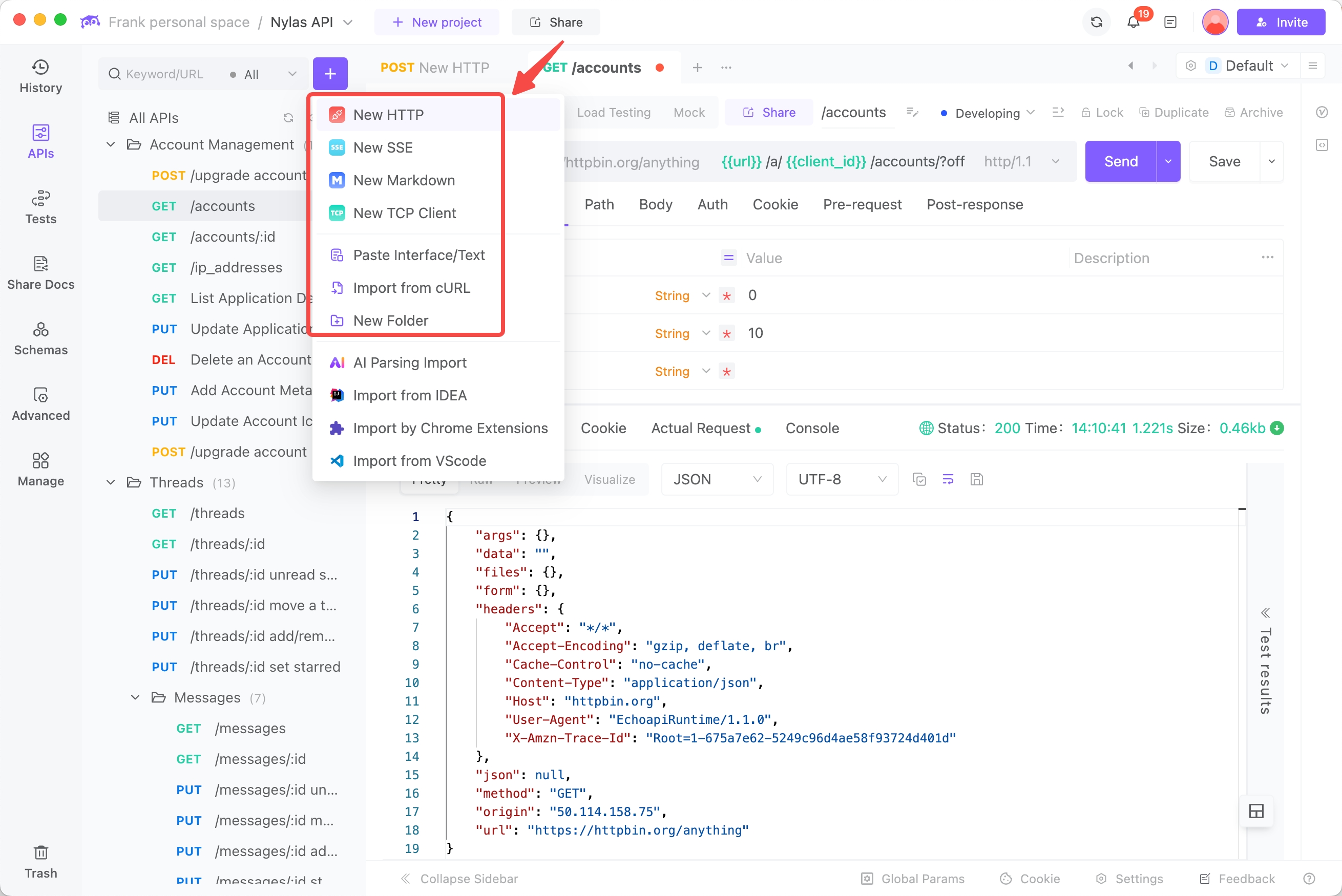
2. Set the Request Method to POST
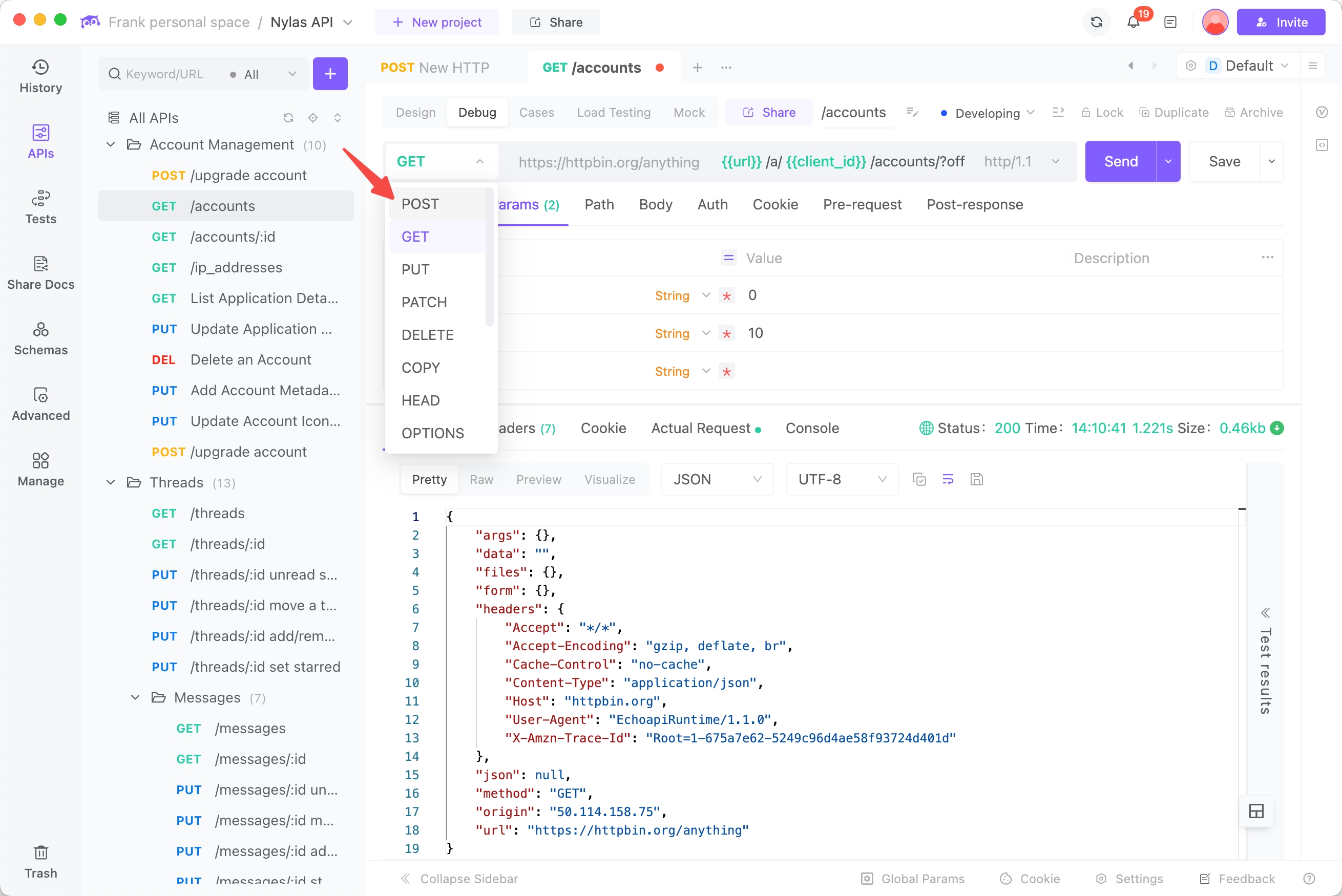
3. Enter the URL of the Resource to Update, Set Additional Headers or Parameters, and Click the "Send" Button to Submit the Request
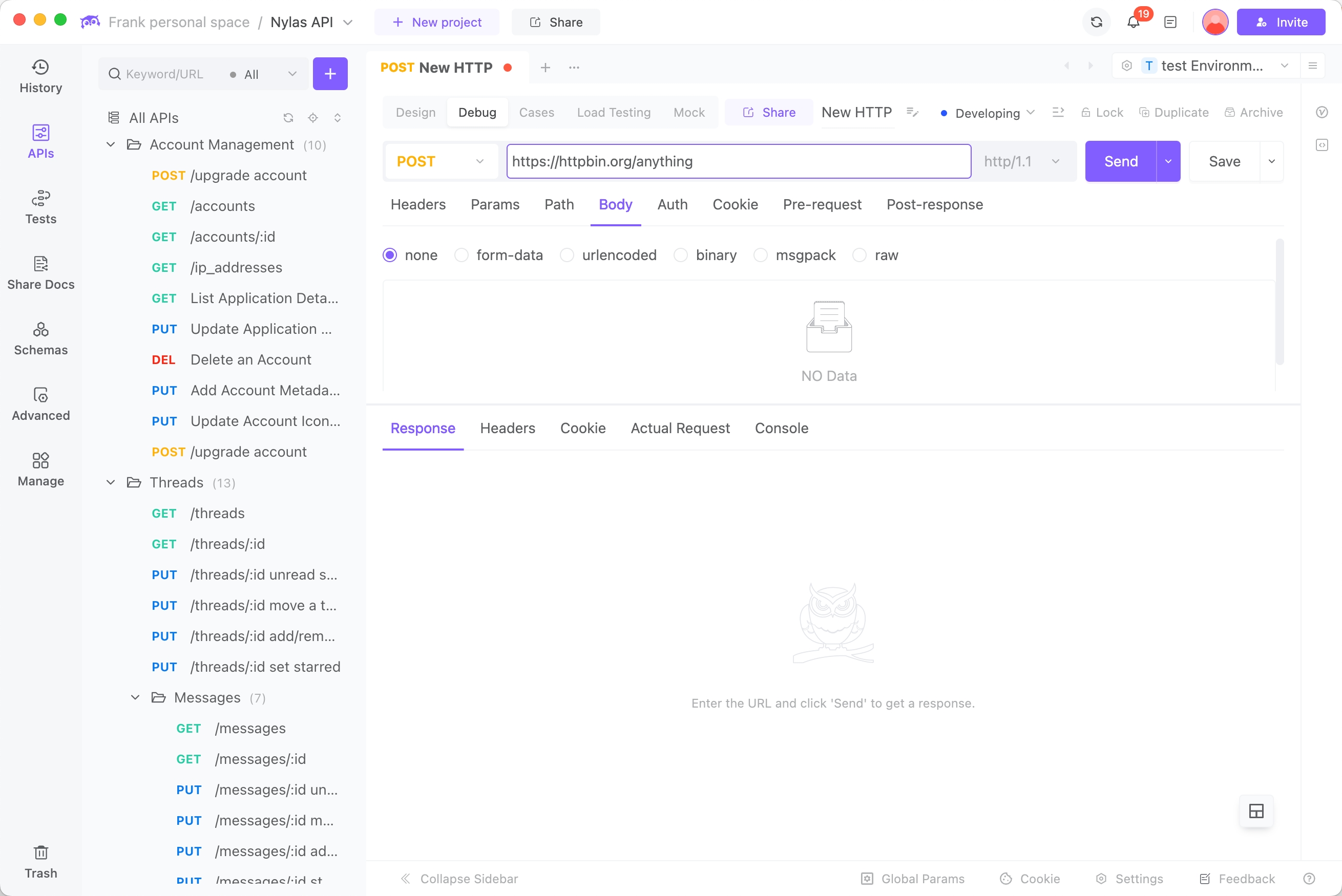
4. Verify That the Response is as Expected
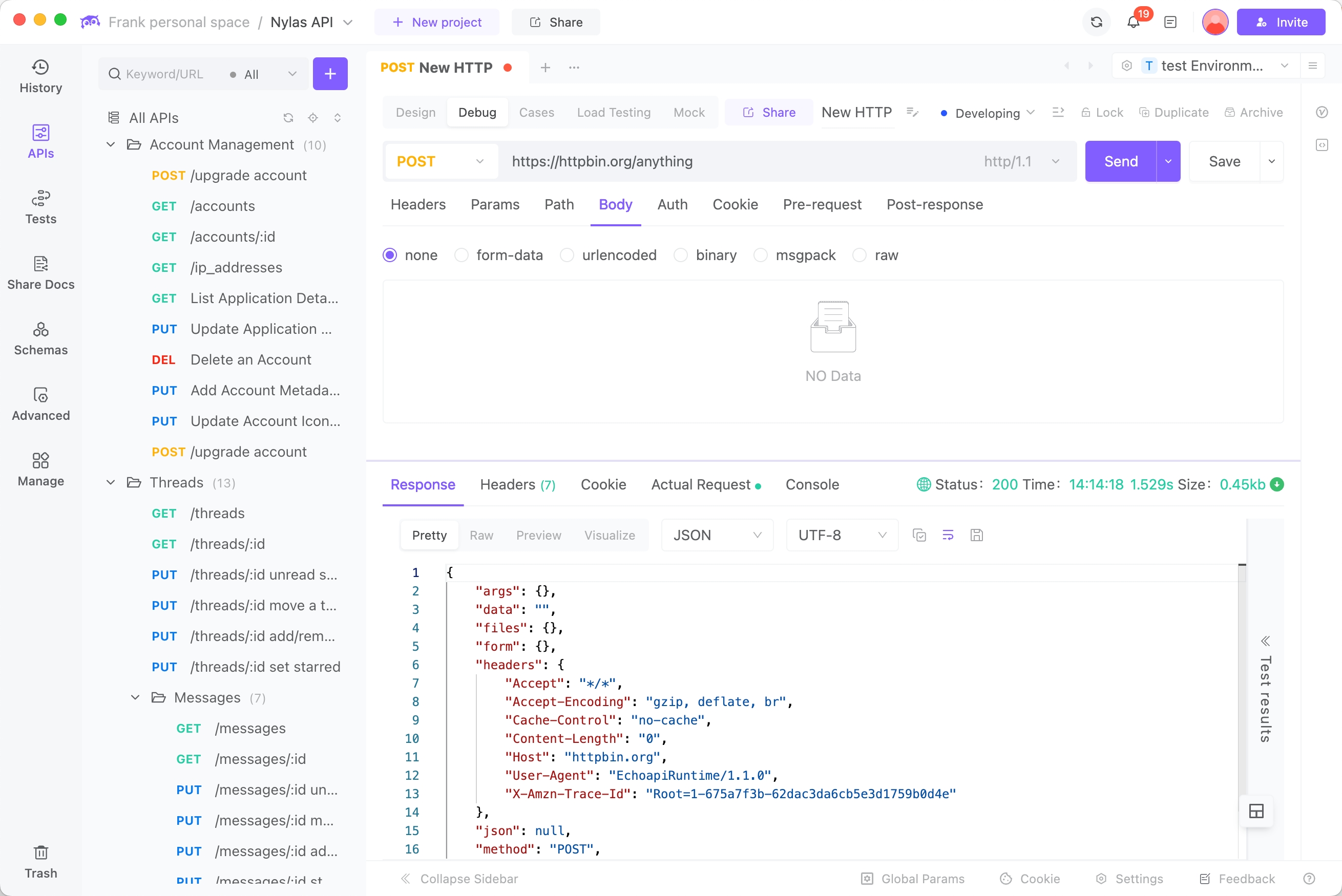
7. Best Practices for POST Requests
When sending POST requests, it's important to follow best practices to ensure your APIs are secure, efficient, and user-friendly. Here are some best practices to consider:
- Use JSON for Data Transfer: JSON is the standard for data transfer. It's widely supported by many frameworks and easy to use.
- API Security: Use HTTPS to encrypt data during transfer. Protect sensitive information with authentication and authorization strategies.
- Graceful Error Handling: Return standard HTTP status codes and clear error messages to indicate what went wrong to the client.
- Support Filtering, Sorting, and Pagination: Enable clients to retrieve only the data they need, improving the API's usability.
- Data Caching: Use caching to reduce server load and decrease response times.
- API Versioning: Maintain different versions of your API, allowing clients to migrate to new versions at their own pace.
- Input Validation: Always validate and sanitize inputs to protect against SQL injection and other attacks.
- API Documentation: Clearly document your API, including expected request and response formats, to make integration easier for clients.
- Use Appropriate Status Codes: Use the correct HTTP status codes to indicate the result of the request. For example, use "201 Created" for a successful POST request that creates a resource.
- Avoid Overloading Query Parameters: Use query parameters for non-sensitive metadata, and send primary request data and sensitive information in the request body.
Following these practices will help you build robust and user-friendly APIs.
Conclusion
Sending POST requests in Python is a fundamental skill for developers working with APIs. By leveraging the requests
library, you can efficiently interact with web services, submit form data, and handle server responses. Adhering to best practices—such as using JSON for data transfer, securing your APIs, testing POST requests with tools like EchoAPI, and validating inputs—ensures that your applications are robust and secure.
With these tools and techniques, you can seamlessly integrate Python into your web development projects and maximize the power of HTTP communication.