Complete List of API Authentication Methods: From Basic Auth to OAuth2 and JWT
API authentication is crucial for protecting application data. Understanding the principles, use cases, and implementation of authentication methods ranging from Basic Auth to OAuth2 and JWT helps developers choose the right approach to ensure the security and reliability of APIs.
Imagine leaving your house every morning with your front door wide open, a big neon sign flashing: “Free Wi-Fi, Free Snacks, No Security Cameras!”
Sounds ridiculous, right?
Well, that’s exactly what you're doing if you build an API without proper authentication.
APIs are the front doors to your app’s most precious data—user profiles, billing info, cat memes-in-progress—and guess what? The internet is basically one big digital neighborhood where not everyone is just out walking their dog.
Without proper locks, keys, and ID checks, your API becomes a buffet for bots, bad actors, and that one coworker who "accidentally" drops your staging token into production.
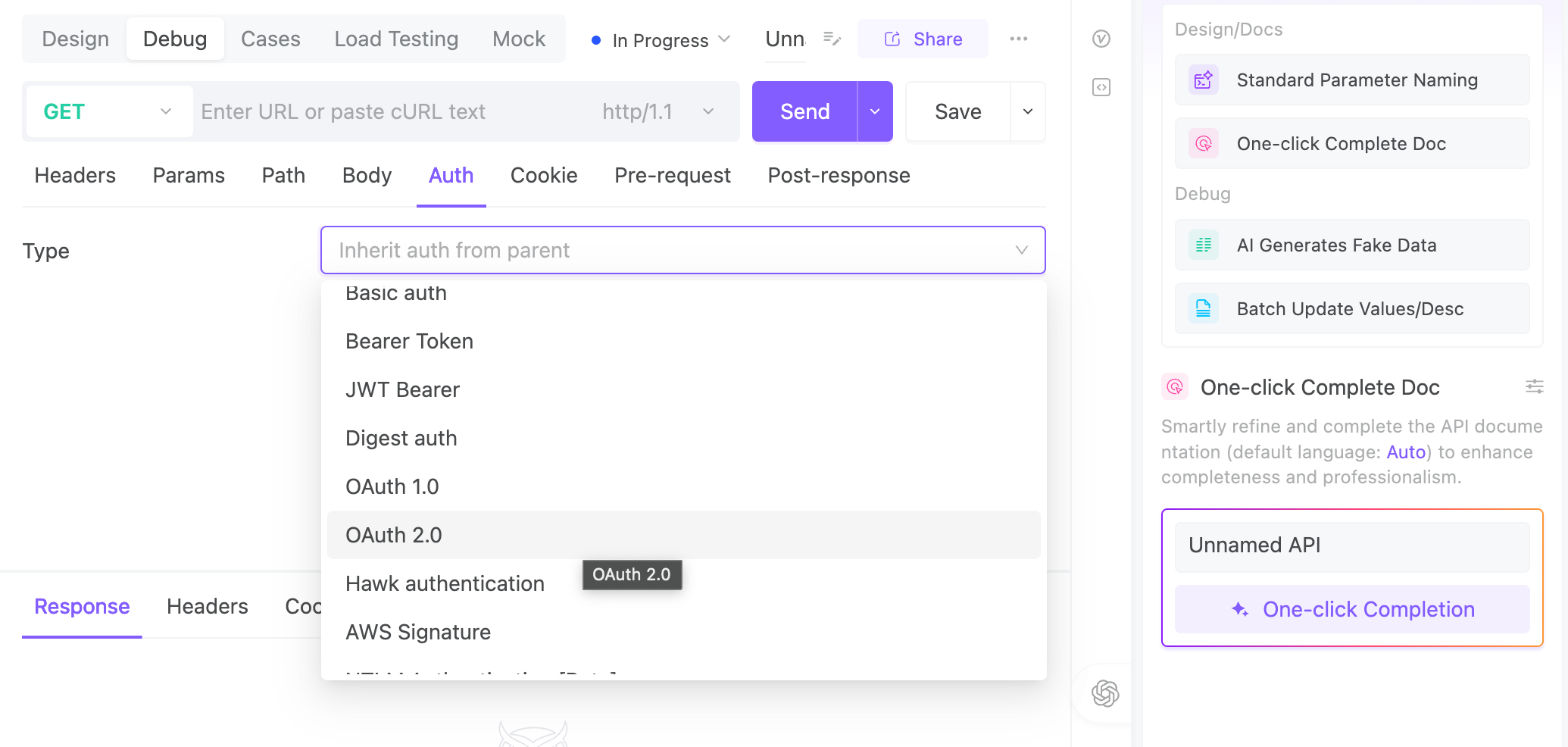
So in this guide, we’re gonna break down the wild world of API authentication:
From simple ID-card-style logins like Basic Auth to James Bond-level encrypted signatures like AWS Signature V4.
We’ll show you what to use, when to use it, and most importantly—how not to accidentally turn your API into an all-you-can-eat hacker buffet.
Strap in. We’re going full Fort Knox on your endpoints.
The Wild World of API Authentication
1. The Cool Kids’ Club: Modern Auth Methods (Use these if you're building anything from this decade)
Auth Method | When to Use | TL;DR |
---|---|---|
Bearer Token | Logged-in users, OAuth 2 access tokens | The gym card of auth – flash it and you’re in |
JWT Bearer | SSO, Microservices | A smart badge – comes with your name, photo, birthday |
OAuth 2.0 | Third-party login (GitHub, Google...) | The bouncer asks your rich friend (Google) if you’re cool enough to get in |
2. The “Old but Gold” Gang: Basic and Legacy Auth (Best for internal use or MVPs your boss demanded yesterday)
Auth Method | When to Use | TL;DR |
---|---|---|
Basic Auth | Internal tools, automation scripts | "Hi, I'm John, room 502" — sends your creds in Base64, not encryption |
Digest Auth | Old systems, simple security | Like whispering your password through a voice modulator |
API Key | Public APIs, user quota tracking | Your hotel keycard — easy to copy, but gets the job done |
3. The Fort Knox Gang: Signed & Sealed Security (If you’re paranoid or in fintech)
Auth Method | When to Use | TL;DR |
---|---|---|
OAuth 1.0 | Twitter v1, legacy APIs | Bureaucracy at its finest — signature, timestamp, nonce, the works |
Hawk | Secure REST-to-REST comms | Like signing every delivery to prove it wasn’t tampered with |
AWS Signature | Calling AWS services (S3, Lambda...) | Requires secret decoder ring, timestamp voodoo, and AWS magic |
Akamai EdgeGrid | APIs behind the Akamai fortress | The CDN’s iron gate — not getting in without the sacred scroll (signed headers) |
4. Internal Affairs: Proprietary and Enterprise Auth (For corp-y setups and Windows nostalgia)
Auth Method | When to Use | TL;DR |
---|---|---|
NTLM Authentication | Intranet, Windows enterprise land | Swipe your corporate badge, say hi to the receptionist (Active Directory) |
ASAP (Atlassian) | Jira, Confluence, CI/CD in Atlassian stack | Atlassian’s internal VIP pass, JWT-based and fast |
5. Testing Tools & Dev Shortcuts (Because typing tokens 100x sucks)
Auth Method | When to Use | TL;DR |
---|---|---|
Inherit from parent | API collection testing | Like wearing a mall-wide wristband – one setup, many shops |
No Auth | Public APIs, early dev stage | “Come one, come all” – no ID required |
Auth Method Deep Dive – With Metaphors
1. Basic Auth – The Classic Username & Password
Analogy: Like telling a security guard, “I’m John from apartment 502,” and they let you in.
Key Features:
- Sends your username and password (Base64-encoded) with each request
- Super simple, but not secure—credentials can be easily intercepted
When to use: In trusted environments (e.g. internal networks or testing)
Best for: Internal systems, small team tools
Request Example:
GET /api/data HTTP/1.1
Authorization: Basic am9objpwYXNzd29yZA== # Base64 of john:password
Python Example:
import requests
from requests.auth import HTTPBasicAuth
requests.get('https://api.example.com/data', auth=HTTPBasicAuth('john', 'password'))
2. Bearer Token – A Simple Access Pass
Analogy: Like using a gym membership card—no one checks your name, just the card’s validity.
Key Features:
- Token represents your session
- Doesn’t carry user info—just proves you’re authorized
When to use: After login, to access user-specific data
Best for: Authenticated API access after login
Request Example:
GET /api/profile HTTP/1.1
Authorization: Bearer eyJhbGciOiJIUzI1NiIsIn...
JavaScript Example (Frontend):
fetch('/api/profile', {
headers: {
'Authorization': 'Bearer YOUR_TOKEN_HERE'
}
})
3. JWT Bearer – A Smart ID Badge
Analogy: Like an ID card that shows your name, photo, and role—not just a pass, it carries info.
Key Features:
- Self-contained token with user data (JSON Web Token)
- No need to hit the database every time
When to use: For microservices, SSO, stateless apps
Best for: Microservices, Single Sign-On
Token Payload:
{
"sub": "user123",
"role": "admin",
"exp": 1710000000
}
Node.js Example:
const jwt = require('jsonwebtoken');
const token = jwt.sign({ user: 'john' }, 'secret', { expiresIn: '1h' });
fetch('/api/data', {
headers: {
Authorization: `Bearer ${token}`
}
});
4. API Key – A Hotel Room Key
Analogy: You need a key card to get into your hotel room.
Key Features:
- A unique string to identify the caller
- Can be passed via header, query, or body
When to use: For simple access control or usage tracking
Best for: Public APIs, platform usage tracking
Header Example:
GET /api/news HTTP/1.1
x-api-key: abc123456789
Query String Example:
GET /api/news?apikey=abc123456789
5. OAuth 2.0 – Standard Third-Party Login
Analogy: You log into Paypal with your Google account—Google vouches for your identity.
Key Features:
- For delegated access to user data
- Multiple flows supported (auth code, password, client credentials, etc.)
When to use: Social logins or inter-company integrations
Best for: Third-party login and delegated access
Simplified Auth Code Flow:
- User is redirected to authorization page
- Gets back a
code
- Server exchanges
code
for access token:
POST https://oauth.example.com/token
Content-Type: application/x-www-form-urlencoded
grant_type=authorization_code&code=abc123&client_id=xxx&client_secret=yyy
- Use token to access data:
GET /user/profile
Authorization: Bearer ACCESS_TOKEN_HERE
6. OAuth 1.0 – The Old-School Method
Analogy: You need a personal signature and a company stamp—extra steps for integrity.
Key Features:
- Request must be signed (Signature)
- Secure but complex
When to use: When the API only supports OAuth 1.0 (e.g., Twitter)
Best for: Legacy APIs like Twitter
Authorization Header:
Authorization: OAuth oauth_consumer_key="key",
oauth_nonce="random",
oauth_signature="hmac_sha1",
...
Python Example:
from requests_oauthlib import OAuth1
auth = OAuth1('YOUR_KEY', 'YOUR_SECRET', ...)
requests.get('https://api.twitter.com/1.1/statuses/home_timeline.json', auth=auth)
7. Digest Auth – Obfuscated Passwords
Analogy: Like speaking your ID number through a voice changer—more secure than shouting it out.
Key Features:
- More secure than Basic Auth (adds salt and hashing)
- Compatibility issues
When to use: Legacy systems that require username + password but want more security
Best for: Old enterprise backends
Python Example:
from requests.auth import HTTPDigestAuth
requests.get('https://example.com/api', auth=HTTPDigestAuth('user', 'pass'))
8. Hawk – Signed Deliveries
Analogy: Like signing for every delivery, including what's inside—ensures it wasn't tampered with.
Key Features:
- HMAC signatures protect request integrity
- Passwords never exposed
When to use: Inter-service APIs or when you need message integrity
Best for: High-security service-to-service APIs
Node.js Example:
const hawk = require('@hapi/hawk');
const credentials = {
id: 'user-id',
key: 'secret-key',
algorithm: 'sha256'
};
const { header } = hawk.client.header('https://api.example.com/resource', 'GET', { credentials });
fetch('https://api.example.com/resource', {
headers: { Authorization: header }
});
9. AWS Signature – Amazon’s Official Format
Analogy: Visiting AWS HQ? You need their official invitation format.
Key Features:
- Strictly formatted request signatures (with timestamp, headers, region, etc.)
- Mandatory for AWS services
When to use: Interacting with AWS APIs (S3, Lambda, etc.)
Best for: AWS service access
Python Example:
import boto3
s3 = boto3.client('s3', aws_access_key_id='AKIA...', aws_secret_access_key='...')
s3.list_buckets()
AWS SDK handles signature generation for you.
10. NTLM Authentication – Windows Internal Badge
Analogy: You swipe your company ID at the office gate—internal network only.
Key Features:
- Windows domain authentication
- Works with legacy internal systems
When to use: Enterprise apps in Microsoft environments
Best for: Internal Windows systems
Python Example:
from requests_ntlm import HttpNtlmAuth
requests.get("http://windows-server.local", auth=HttpNtlmAuth('DOMAIN\\user', 'password'))
11. Akamai EdgeGrid – Secure Gatekeeping at Scale
Analogy: Before airport security lets you through, your pass must match their secure format.
Key Features:
- Akamai-specific signing mechanism
- Ensures secure, large-scale traffic handling
When to use: Calling APIs protected by Akamai
Best for: Enterprise APIs behind Akamai
Authorization Header:
Authorization: EG1-HMAC-SHA256 client_token=xxx;access_token=yyy;timestamp=...;nonce=...
Node.js Example:
const edgegrid = require('akamai-edgegrid');
const eg = new edgegrid('client_token', 'client_secret', 'access_token', 'host');
eg.auth({
path: '/endpoint',
method: 'GET'
});
12. ASAP (Atlassian Service Auth Protocol) – Internal Employee Badge
Analogy: Moving between departments inside a company, your staff ID grants access automatically.
Key Features:
- Atlassian’s JWT-based service-to-service auth
- Signed with private key
When to use: Internal calls within Atlassian ecosystem or microservices
Best for: Atlassian internal services, CI/CD pipelines
Authorization Example:
Authorization: Bearer eyJraWQiOiJhc2FwS2V5IiwidHlwIjoiSldUI...
13. Inherit from Parent – One Login to Rule Them All
Analogy: Enter the main mall with a pass, and all the stores inside let you through without rechecking.
Key Features:
- Child requests inherit auth from parent config
When to use: Tools like EchoAPI, where you test many endpoints with shared auth
Best for: API testing tools (EchoAPI collections)
How it works: Set auth once at the collection level, child requests reuse it
Parent collection sets Bearer Token → All requests inherit automatically
14. No Auth – Open for All
Analogy: Like a public park—no ID, no ticket, just walk in.
Key Features:
- No authentication needed
When to use: Public data, development stubs, or health check endpoints
Best for: Public APIs, dev environments
GET https://api.weatherapi.com/v1/current.json?q=Beijing
Bonus: Debugging All This Without Crying? Try EchoAPI
Sick of juggling tokens, headers, and cryptic signatures? EchoAPI makes debugging APIs feel like sipping coffee instead of writing 500-line curl scripts.
Real-World Ready: Whether you're calling GitHub or an internal legacy blob, EchoAPI has your back
Unified Testing: One place to test everything from Bearer to AWS Signature
Enterprise-Approved: From NTLM to Akamai EdgeGrid, it's got the big boys covered
Speedy Debugging: Simulate logins, auth flows, service calls – no more manual header copy-paste hell
If you want to learn more about encryption methods, feel free to search on the EchoAPI's blog.
More related articles:
Learn More:
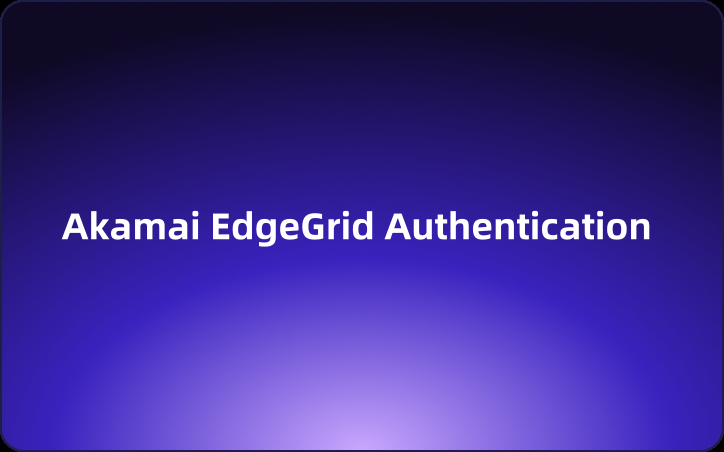
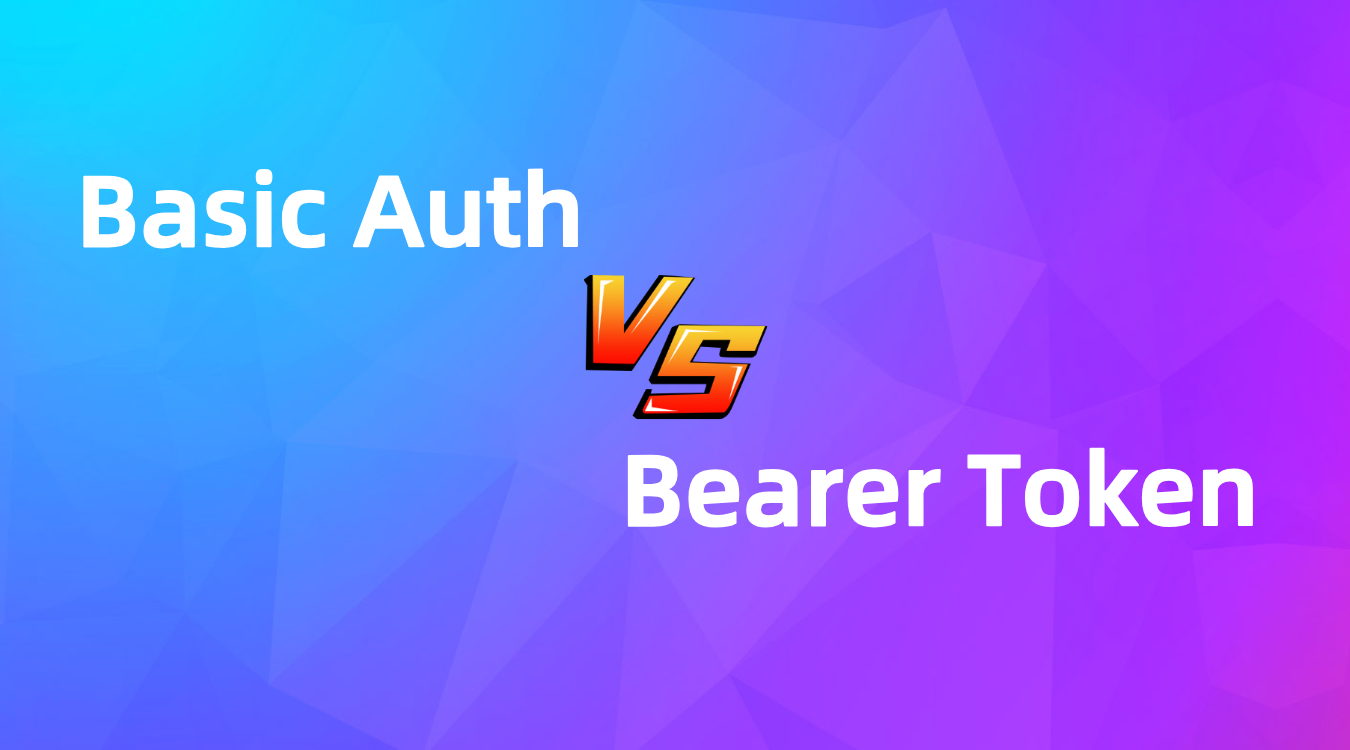
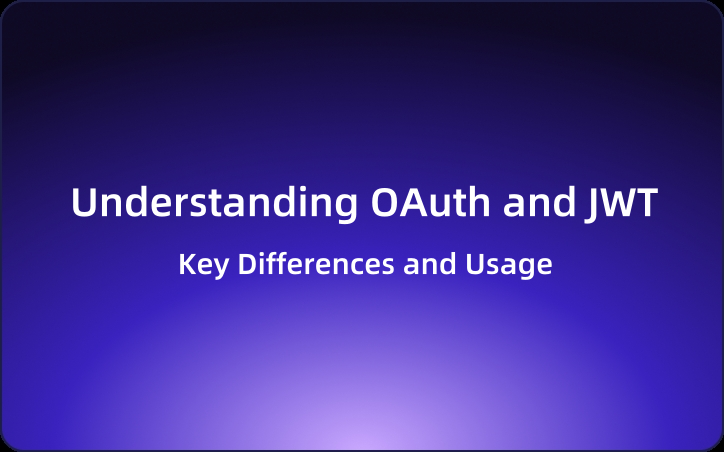