Behind Every API: What Status Codes, Error Codes, and Assertions Really Mean
This article delves into API status codes, error codes, and assertions, and how they determine the success or failure of API calls. With these elements, developers can better diagnose and fix issues when interacting with APIs.
"HTTP status code 200? Sweet! Wait… why am I getting an error message?!"
Welcome to the deceptively tricky world of status codes and error codes—where success isn't always what it seems, and a green light doesn’t mean you’ve reached your destination.
This guide is your roadmap through the maze:
- First stop: What the heck is a status code, really? (Hint: it’s like a delivery guy yelling “Package delivered!” or “Address not found!” at your door.)
- Then we dive into error codes—those cryptic little gremlins in the response body that say, “Nope, your request technically worked, but it actually failed.”
- And finally, meet your new best friend: assertions. Think of them as your API's lie detector test—sniffing out hidden failures even when everything looks fine.
By the end, you’ll know how to tell if your API is truly behaving, or just faking it like a student who says “present” in class while sneaking out the window.
First Stop: What the Is a Status Code?
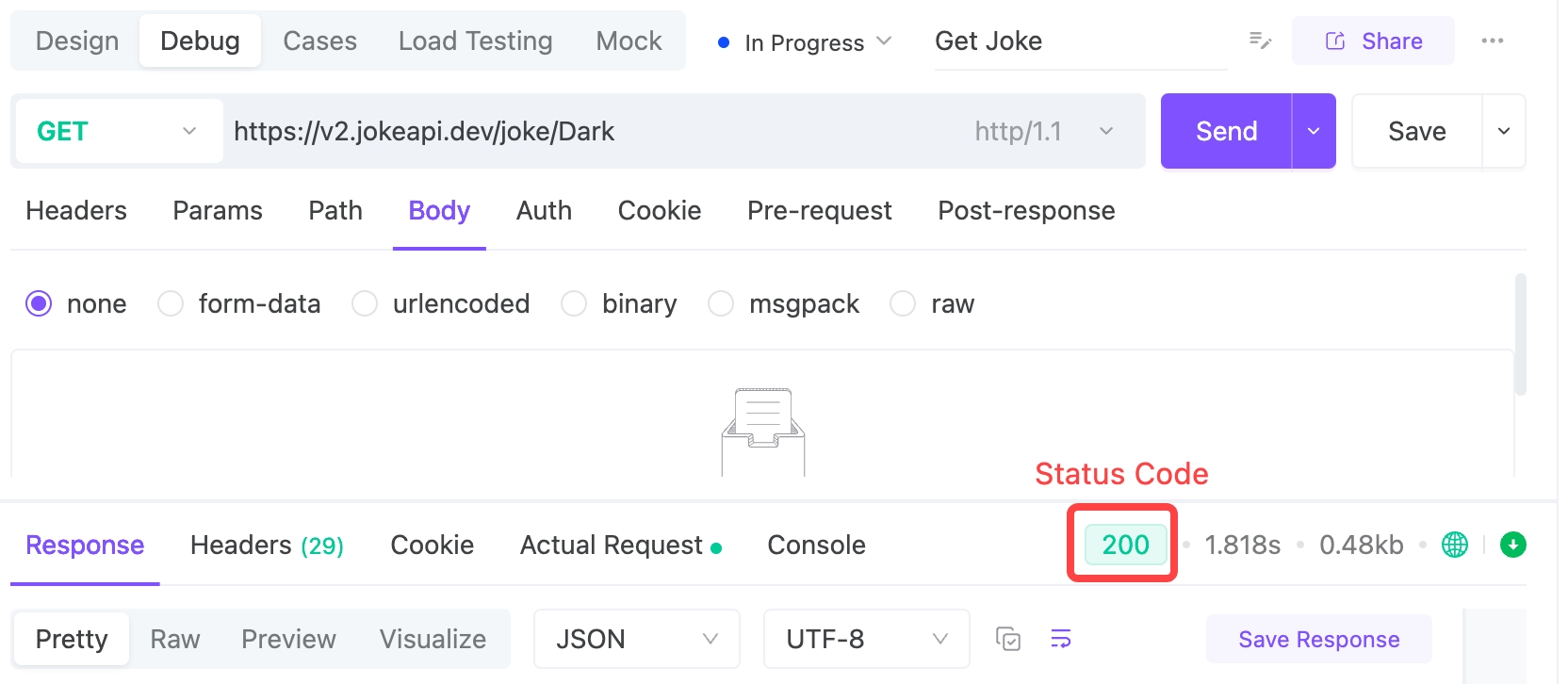
Imagine sending a package. The courier’s updates are brutally blunt:
- “Delivered.” (200)
- “Address not found.” (404)
- “You’re not allowed to ship this.” (403)
- “Our warehouse exploded.” (500)
That's what HTTP status codes do — your friendly (or not-so-friendly) server yelling back how your request went on a technical level.
Common HTTP Status Codes:
Status Code | Meaning | Real-World Example |
---|---|---|
200 | OK, request succeeded | Login successful, data fetched |
201 | Created | New user or order created |
204 | No Content | Deletion worked, no need to reply |
400 | Bad Request | Missing fields, invalid format |
401 | Unauthorized | Missing or expired token |
403 | Forbidden | You’re not admin, buddy |
404 | Not Found | User ID? Yeah, that doesn’t exist |
500 | Internal Server Error | DB meltdown, code panic, etc. |
💡 Think of status codes as traffic lights — they tell you if the highway is open, but not what’s in the trunk.
Second Stop: So... What Are Error Codes?
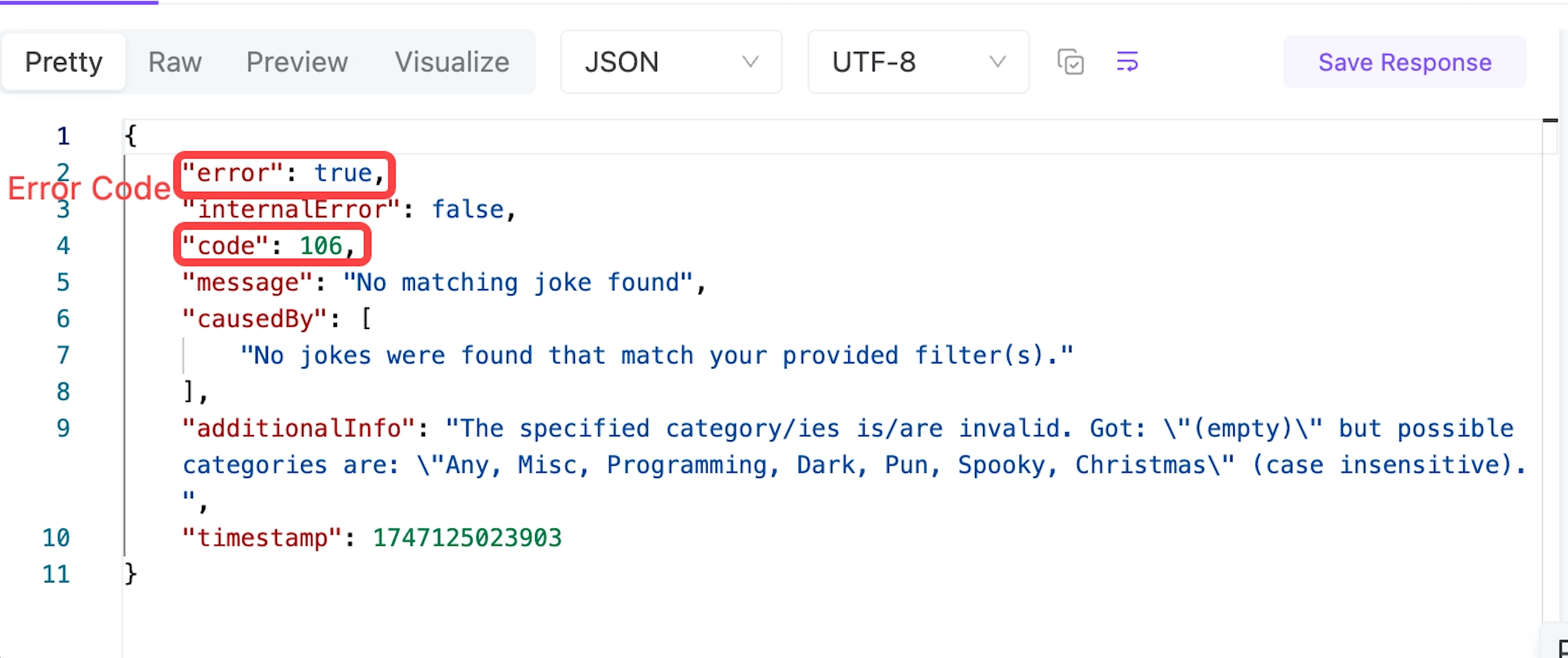
You call an API. Status code is 200. You nod. “Nice, all green.”
You peek at the response body — and boom:
{
"error": true,
"internalError": false,
"code": 106,
"message": "No matching joke found",
"causedBy": [
"No jokes were found that match your provided filter(s)."
]
}
Wait, what?! “I thought 200 meant success!”
Plot twist: it technically did.
Truth Bomb: HTTP Status ≠Business Success
HTTP status codes (200, 404) are just the envelope.
They tell you the server received your request and responded — not whether your actual request made any sense.
- âś…
status code = 200
: Congrats! The server’s alive and responded. - ❌
code = INVALID_REQUEST
: Oops. Your data’s wrong, your business logic failed.
HTTP status is the bouncer. Business error codes are the hiring manager. You got in the door… but got rejected anyway.
So, What’s an Error Code Then?
Error codes are custom-made by backend devs to represent business logic failures.
Think: login fails, product is out of stock, credit card is expired — that kind of real-life mess.
Different teams define different codes — but here’s the usual suspects:
Common Business Error Codes
Error Code | Seen In | Meaning |
---|---|---|
INVALID_REQUEST |
Generic | Missing fields, wrong types |
UNAUTHORIZED |
Generic | User not logged in, token invalid |
FORBIDDEN |
Generic | You don't have permission |
NOT_FOUND |
Generic | Resource doesn’t exist |
CARD_EXPIRED |
PayPal | Your card’s way past its bedtime |
INSUFFICIENT_FUNDS |
PayPal | Not enough money, time to panic |
INVALID_ARGUMENT |
Google APIs | Argument out of range, or wrong format |
ALREADY_EXISTS |
Google APIs | Resource already exists |
PERMISSION_DENIED |
Google APIs | You’re not allowed to access that |
đź§ľ Example Error Responses
Stripe-style:
{
"error": {
"type": "invalid_request_error",
"code": "resource_missing",
"message": "No such customer: 'cus_123'"
}
}
PayPal-style:
{
"name": "CARD_EXPIRED",
"message": "The card is expired.",
"details": [
{
"field": "/payment_source/card/expiry",
"issue": "CARD_EXPIRED"
}
]
}
But What If There’s No Standard Error Code?
Sometimes the backend team just… wings it. Or there's no shared doc.
Here’s what you can do:
- Ask your backend: “Yo, got a global error code doc?”
- Write assertions to catch recurring issues.
- Push for a proper error code strategy in your team — trust me, future-you will thank you.
⚡ HTTP status tells you if the power’s on. Error code tells you if the lights actually turned on.
Status Code vs Error Code: Know the Layers
Layer | Defined By | Purpose | Example |
---|---|---|---|
Status Code | HTTP Standard (IETF) | Did the request technically succeed? | 200, 404, 500 |
Error Code | You / Your Company | Did the business logic succeed? | CARD_EXPIRED, PERMISSION_DENIED |
💡 Status code = “Did the elevator work?”
Error code = “Did you get to the right floor?”
Final Stop: Why Assertions Are Your Secret Weapon
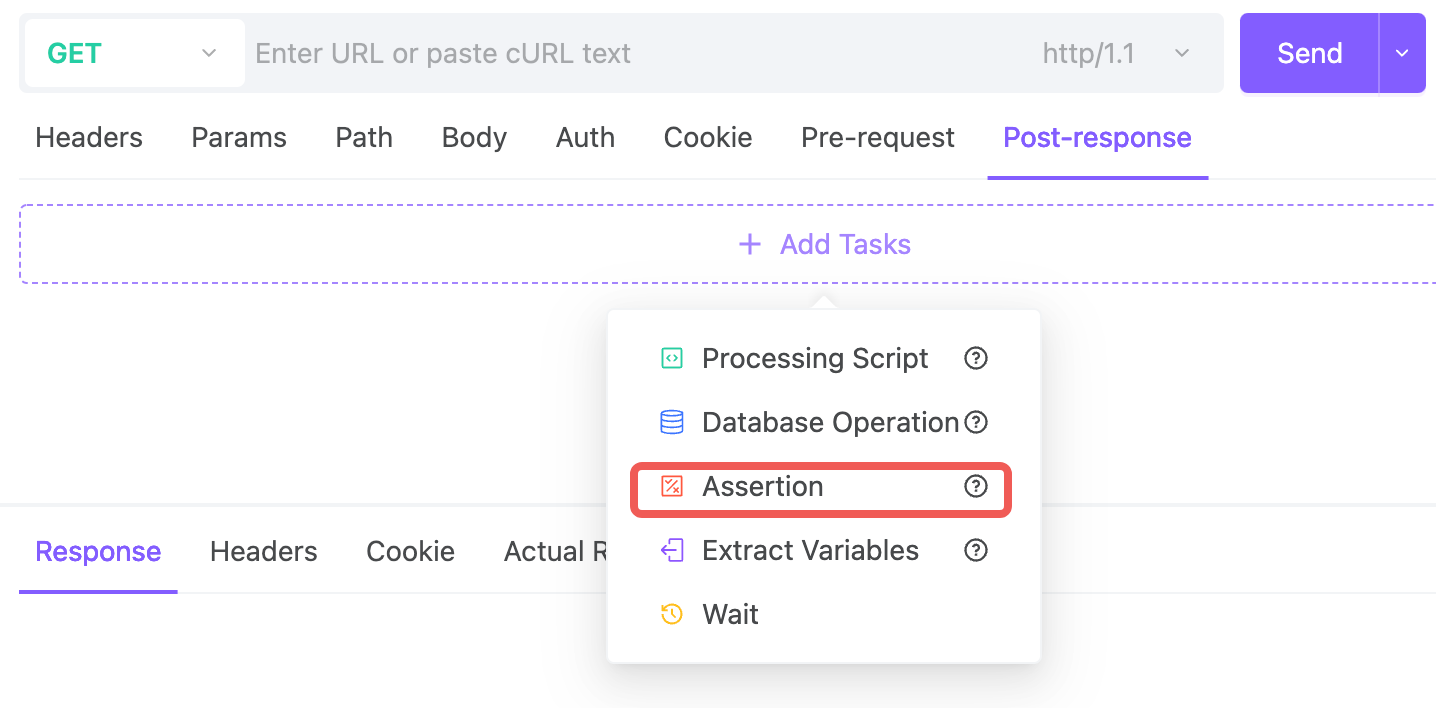
Why Assertions?
Because…
- You can’t trust status codes alone
- You need to auto-catch silent failures
- You want to detect regressions fast
- And let’s be real: eyeballing JSON is a pain
How EchoAPI Helps You Assert Like a Pro
Set up custom assertion rules like:
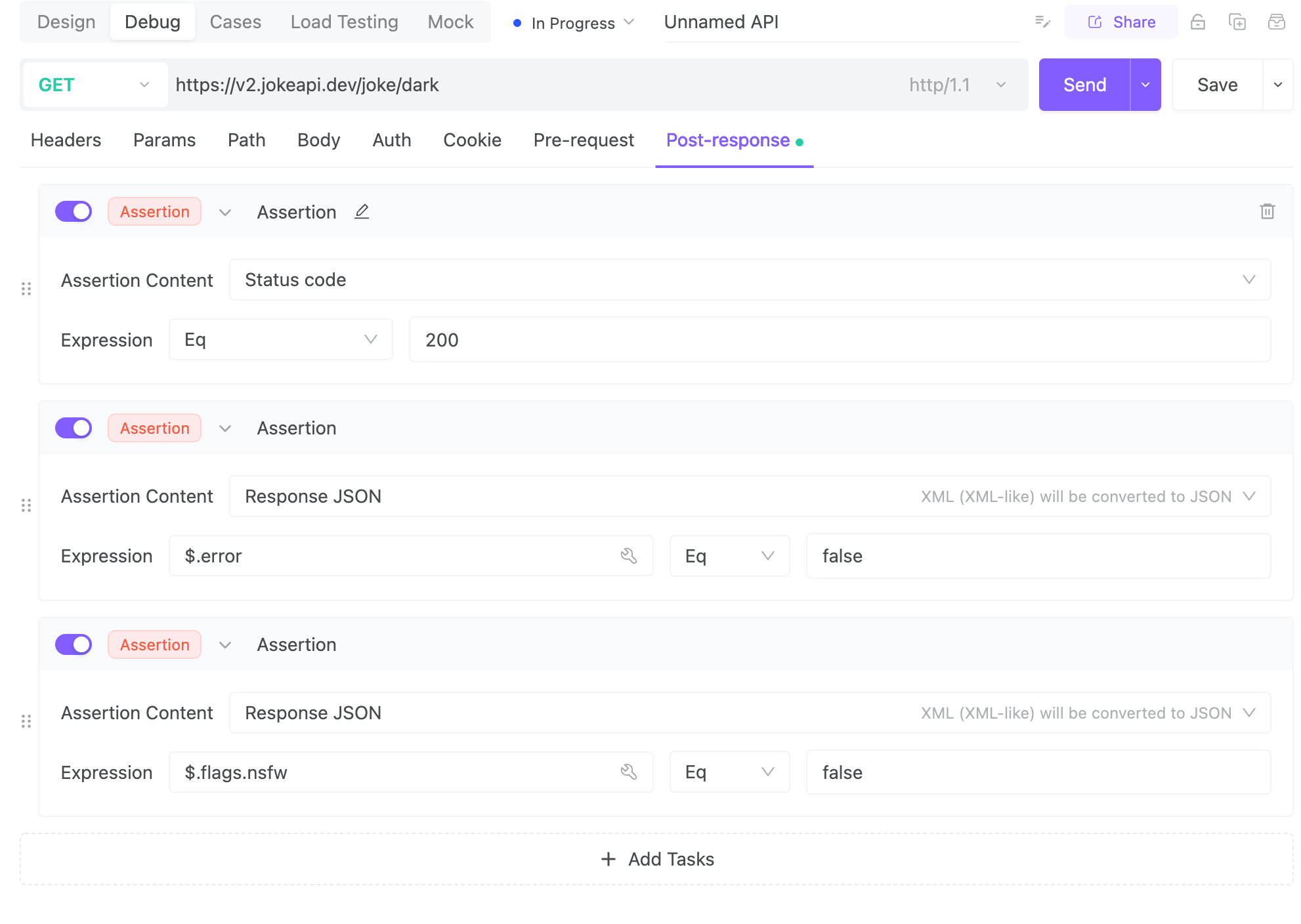
Get instant visual feedback on success or failure:
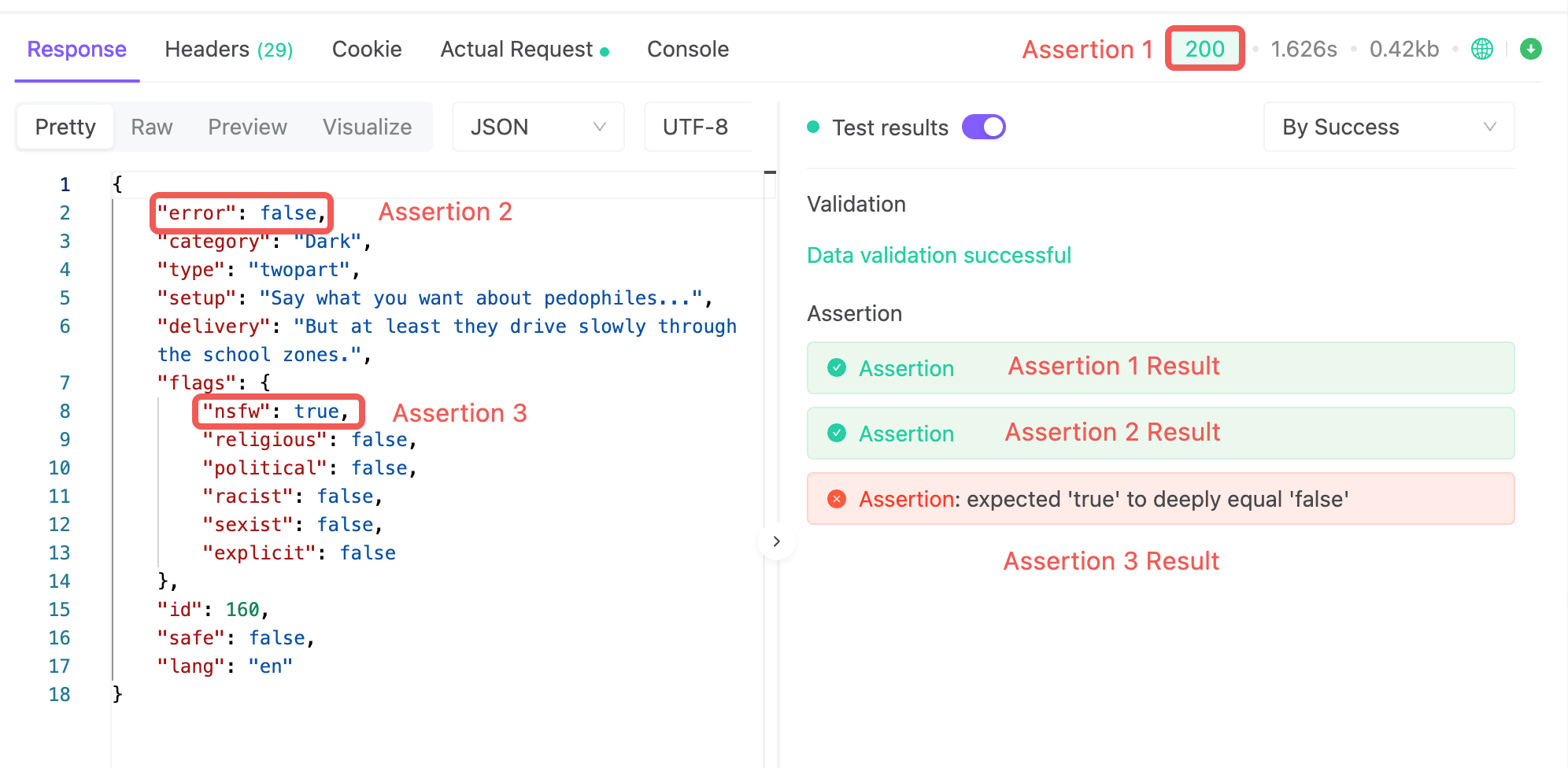
Why Assertions Are Your API’s Bodyguard
Assertions are like x-ray goggles for your APIs. They catch:
1. Silent failures behind green status codes
Just because it says 200 OK
doesn’t mean your login succeeded.
Assertions let you verify key fields like error = false
, data != null
, or code == 0
.
2. Mass testing without losing your sanity
Running 50 APIs manually is a nightmare.
With assertions + automation:
- Run tests in bulk
- See exactly which step failed, and why
- Plug it into CI/CD, get alerts the moment things break
3. Catch sneaky field name changes
Your backend quietly renames userName
to username
.
Looks like a 200
, smells like success — but your frontend breaks.
Assertion catches it.
You laugh while others cry.
Assertions = API fire alarms + lie detectors, all in one.
Without Assertions vs With Assertions
Feature | Just Check Status | Use Assertions âś… |
---|---|---|
Detect business logic failures | ❌ Nope | ✅ Yup |
Auto-detect errors | ❌ Manual only | ✅ Automated |
Run batch tests | ❌ Tedious | ✅ One click |
Detect field name changes | ❌ Gotta squint | ✅ Instant alert |
Validate before release | ❌ Hope for best | ✅ CI approved |
Conclusion
Status codes are the doorman — they tell you if you got into the building.
Error codes are the receptionist — they tell you if your appointment was successful.
Assertions are the bodyguard — they make sure nobody lies to you.