API Parameter Guide: How to Customize Your API Like a Bubble Tea Order
This article offers a comprehensive guide on what API parameters are and how to use them effectively, catering to both beginners and advanced users. By understanding the proper way to use them, you can improve data accuracy, streamline development, and transform your interaction with APIs.
You pop open your browser like a seasoned hacker, and type in what looks like an incantation from a cyberpunk spellbook:
GET /api/teas?flavor=oolong&toppings=boba,sago&sweetness=half&ice=less&size=medium
Looks like you're trying to hack into NASA?
But actuallyânope! You're just ordering bubble tea... in API language.
Letâs break this down:
/api/teas
: You're ordering tea.flavor=oolong
: You want the oolong flavor.toppings=boba,sago
: Add boba and sago (yes, you can stack âem).sweetness=half
: Half sugar, please.ice=less
: Less ice, because youâre a purist.size=medium
: Medium size, the Goldilocks option.
Itâs like you're talking to a tea-making robot in the API cafĂ©:
"Yo, one medium oolong milk tea, half sugar, less ice, with boba and sago. Thanks~"
The server reads your order slip, nods silently, and assembles your custom cup of teaâor, in reality, returns exactly the data you asked for.
That little slip of paper carrying all your preferences? Thatâs the star of todayâs show: params.
What Are Params, and What Are They Good For?
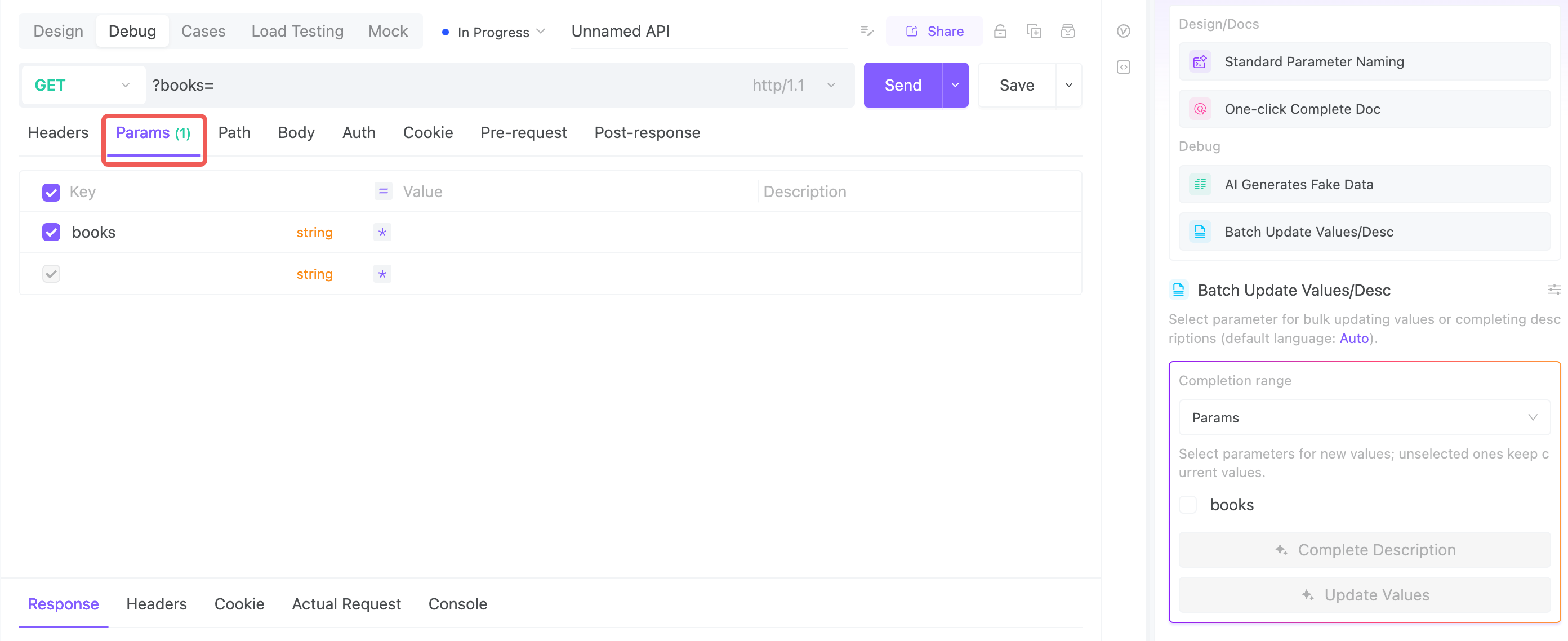
Params are how you tell the server: âHey, I want this resource, but with some extra seasoning.â
If you hit /api/books
, itâs like saying:
âShow me the books.â
But if you slap on ?category=history&page=2&limit=10
, you're basically saying:
âGive me history books, page 2, 10 books per page.â
Think of it like the waiter asking:
âAny special requests?â
Params are your way of sliding over a post-it with all the specifics.
Are Params Only for URLs?
Heck no! Params are like condimentsâtheyâre not just sprinkled on top. They can be stirred into the soup, folded into the dough, or hidden in a secret sauce packet.
Besides the classic URL version:
- POST/PUT requests often carry them in the body
- RESTful routes bake them into the path (
/books/:id
) - HTML forms tuck them into x-www-form-urlencoded or form-data
- Sometimes theyâre even stuffed into headers or cookies
If youâre passing extra info, youâre using paramsâwhether you know it or not.
The Many Faces of Params
Params arenât always one neat little form field. Theyâre master shape-shifters. Depending on the request style, they wear different disguisesâbut they all serve the same purpose:
Telling the server what you really want.
Letâs check out their most popular outfits:
Query Params â The Classic Toppings List
This is the chill casualwear of params. They show up right after a ?
, with each key-value pair separated by &
.
Ordering tea with query params looks like:
GET /api/teas?flavor=oolong&ice=none&sweetness=half&size=medium&toppings=boba
Itâs like walking into a tea shop and saying:
âOne oolong milk tea, no ice, half sugar, medium size, with boba!â
â Best for:
- Filtering lists (e.g.,
flavor=matcha
) - Pagination (
page=2&limit=10
) - Sorting (
sort=price_asc
) - Keyword search (
q=cheesefoam
)
â Perks:
- Easy to cache (URL uniquely identifies the result)
- Copy-paste and debug-friendly
- Lightweight and transparent
Path Params â The Point-and-Order Style
These are baked right into the URL path. Like pointing at the #123 on the menu and saying:
âThat one! Gimme #123!â
Example:
GET /api/teas/123
Here, 123
is the tea ID.
You can even stack them:
GET /api/customers/456/orders/2024
Meaning: âShow me what customer #456 ordered in 2024.â
â Best for:
- Fetching a specific resource (order details, product page)
- Expressing hierarchy (user â order â item)
â Perks:
- Clean and semantic (very RESTful)
- Not ideal for optional filters or long queries
Body Params â The Full Recipe Sheet
This is the VIP treatment. You hand the server a full-blown recipe:
POST /api/teas
{
"flavor": "jasmine",
"size": "large",
"toppings": ["boba", "pudding"],
"sweetness": "quarter",
"ice": "less"
}
The server reads it and goes:
âAh, a large jasmine milk tea with boba and pudding, quarter sugar, less ice? Coming right up!â
â Best for:
- Creating new resources (orders, comments, accounts)
- Submitting structured forms
â Perks:
- Rich, structured, and clean
- Hidden from the URL (more secure)
- Great for POST/PUT/PATCH
- Less cacheable (which is often what you want when modifying data)
Form Params â The Old-School Signup Sheet
This oneâs retro. It mimics good olâ HTML form fields and sends data in key-value format.
Like:
username=milklover&password=iloveboba
Or when uploading files:
Content-Type: multipart/form-data
Itâs your go-to when users upload avatars, receipts, or grandmaâs secret tea recipe.
â Best for:
- Login / registration forms
- Uploading files from web forms
â Perks:
- Works well with HTML forms
- Familiar for older systems
- Not as clean as JSON, but gets the job done
Params Are Just Ways of âOrdering Your Dataâ
- You check boxes on a form: Query Params
- You point at a menu number: Path Params
- You write out custom instructions: Body Params
- You fill out a form with fields: Form Params
Different flavors for different moments, but all pointing to one thing:
âThis is what I want, and how I want it.â
Should You Define Params in Advance?
Yes you should.
Think of it like a chain tea shop needing to standardize their order slips. If everyone makes up their own labels, itâs chaos in the kitchen.
Common disaster scenarios:
- Frontend sends
pageNum
, backend expectspage
- Frontend sends
bookType
, backend looks forcategory
- One typo in a param key and poofâno response, no clue
Without clear rules, everyone codes on assumptions, and ends up in a sad Slack thread titled:
âWhy isnât this working?â
Benefits of Defining Params Early
Having a clear param spec is like giving everyone a laminated menu:
1. Frontend & Backend Stay In Sync
You call it page
, I call it page
, we all stay happy. No "wait, when did that change?" drama.
2. Autogenerated Docs = Happy Devs
Use tools like EchoAPI to write your param schema once, and boom:
- Auto-generated API docs
- Mock Data
3. Debugging and Testing Are Easier
No more âwhy is this blank?â mystery hunts. You know what to send and what to expect.
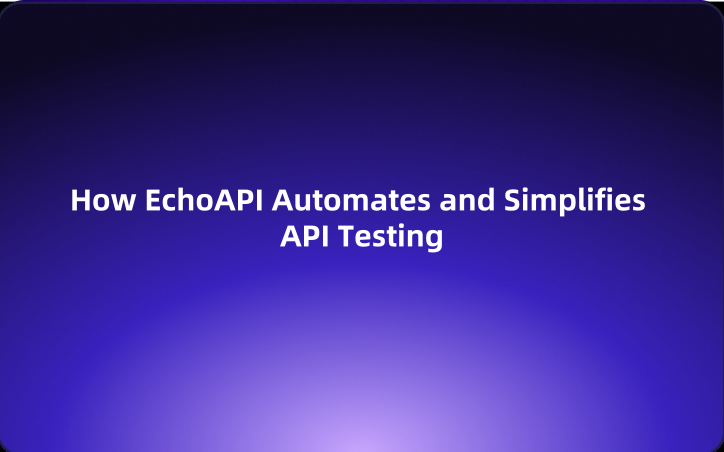
4. Easy Param Validation
Frameworks can auto-check for missing or invalid params and give helpful error messages before things go boom.
5. Cleaner Refactors
Want to rename page
to pageIndex
? If itâs centrally defined, itâs a one-liner fixânot a wild hunt across 47 files.
What Happens If You Donât Define Params?
Simple: total chaos.
- You think the param is
bookType
, the backend is waiting forcategory
- You think pages start at 0, the backend starts at 1
- Frontend changes something, backend yells âBRO WHYâ
- Everyoneâs debugging until 2am, surviving on caffeine and existential dread
Itâs like trying to cook dinner together while speaking different languagesâwith blindfolds on.
Tired of all these chaos? Don't want to write API docs by hand anymore? Sick of copy-pasting the same parameters across endpoints like itâs Groundhog Day?
Say hello to EchoAPI â your ultimate API design assistant that makes documenting, naming, and reusing fields feel as easy (and satisfying) as customizing your favorite bubble tea.
Auto-Generated Docs: Let the Docs Write Themselves
Once you define your API parameters in EchoAPI, it automatically generates beautiful, structured documentation â no more wrestling with Notion, Word, or outdated Swagger files.
Front-end, back-end, QA â everyone stays on the same page. No more Slack threads titled âwhat does this param even do??â
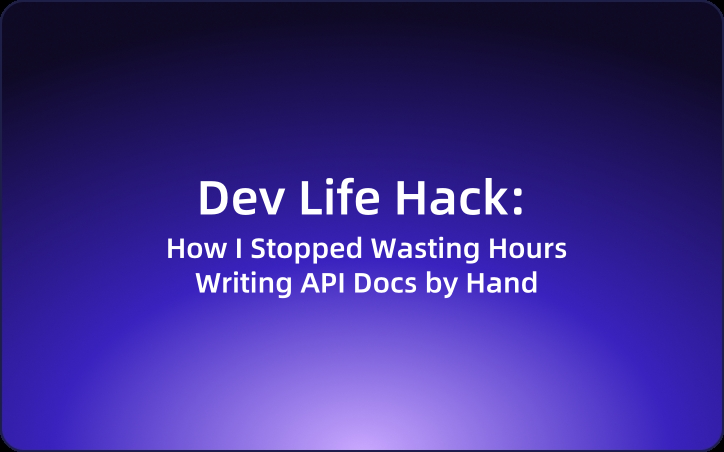
Reusable Field Dictionary: Define Once, Use Everywhere
Ever found yourself defining page
, pageNum
, and page_number
in different endpoints? EchoAPI stops that madness.
It keeps a centralized library of all your fields â names, types, descriptions â so next time youâre building a new endpoint, you can just reuse whatâs already there.
- No more inconsistent naming
- Easy mass updates across the board
- Less typing, more shipping
Itâs like having a personal naming assistant who never forgets how you like your parameters.
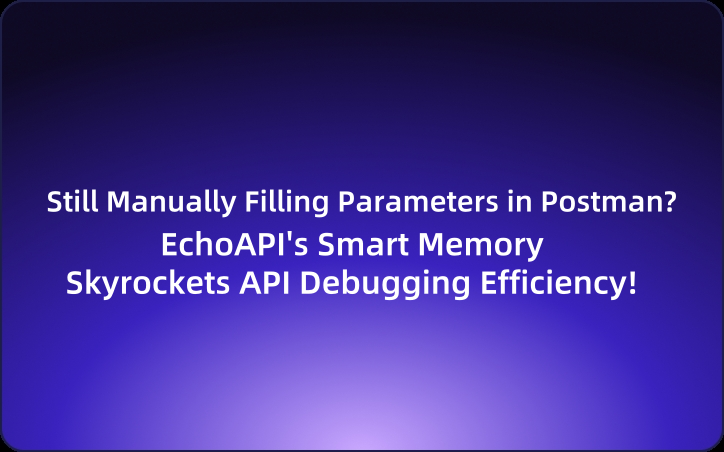
AI-Powered Field Suggestions: Stop Naming Fields Like Itâs 1999
Just type in a description like "userâs birthday" or "time order was placed", and EchoAPIâs AI suggests the perfect field name (birthday
, createdAt
, etc.), complete with type and description.
You donât have to argue over camelCase vs snake_case anymore â EchoAPI helps you stay consistent without breaking a sweat.
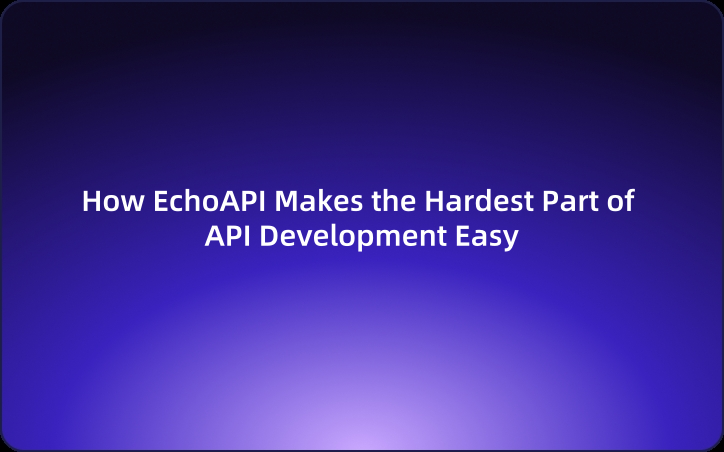
EchoAPI isnât just a tool, itâs your API design sidekick. It helps you:
- Standardize naming conventions
- Eliminate repetitive work
- Avoid miscommunication between teams
- Deliver cleaner, more reliable APIs faster
Whether youâre building a simple endpoint or architecting a full-blown microservice empire â EchoAPI is the boba straw that makes everything flow smoother.
In Conclusion: Params Are the Bubble Tea Stickers of the Internet
They may look small, but params are everywhere. They help you:
- Request data more precisely
- Communicate intent more clearly
- Avoid drowning in magic strings and inconsistent keys
Defining them early, naming them consistently, and managing them wisely.
Thatâs the key to building APIs that are smooth, predictable, and honestly⊠a joy to work with.
When you send a param, youâre not just sending a valueâyouâre placing an order. Youâre making a pact. Youâre building trust between humans and machines.
So next time you hit that endpoint, donât just wing it. Craft your params with the same care you order your bubble tea: clearly, thoughtfully, and maybe with a little boba.
Ready to stop fighting with your API specs and start sipping that sweet, sweet productivity?
Give EchoAPI a spin. Your future self (and your team) will thank you.